11
JulThe map() Function in Python
The Python map() function is a built-in higher-order function that applies a given function to each element in an iterable (such as a list, tuple, or set) and returns an iterator of the resulting values.
In this Python Tutorial, we will explore what the Python map function is, its basic syntax, its use cases with other data structures, and some examples.
What is the map() function?
- The `map()` function is a built-in higher-order function in Python that is used to apply a specific function to each item in an iterable, such as a list, tuple, or set. The function specified is applied to each element individually.
- Instead of returning a list directly (as in some other languages), it returns an iterator of the results, and this iterator can be converted into a list or other collection types if needed.
- `map()` is particularly useful for applying transformations to large datasets efficiently.
- Common use cases include data processing, transforming data, and applying functions to collections of items.
- Syntax typically involves passing the function and the iterable to `map()` like so: `map(function, iterable)`.
- It can be combined with lambda functions for concise inline operations.
- Using `map()` can improve code readability and performance when working with large datasets.
Syntax
Here is the syntax of the map() function in Python
map(function, iterables)
parameters:
- function: Here we write the function we want to apply to each element
- iterable: This represents the iterable whose elements we want to process.
Python map() Function Examples
Example 1
# Python example program for map() function
numbers = [3, 4, 9, 4, 3]
# lambda function defines the squaring operation
squared_numbers = list( map( lambda x : x**2, numbers ))
# print the list of squared numbers
print(squared_numbers)
Output
[9, 16, 81, 16, 9]
Explanation
- numbers is a list of integers: [3, 4, 9, 4, 3].
- map() applies a lambda function that squares each number (x**2).
- list() converts the map object to a list.
- print(squared_numbers) outputs the squared numbers.
Example 2
# Python example program for map() function
temperatures = [0, 5, 15, 20, 25]
# lambda function defines the conversion formula
fahrenheit_temperatures = list(map( lambda x : (9/5)*x + 32, temperatures ))
# print the list of Fahrenheit temperatures
print(fahrenheit_temperatures)
Output
[32.0, 41.0, 59.0, 68.0, 77.0]
Explanation
- temperatures is a list of Celsius values: [0, 5, 15, 20, 25].
- map() applies a lambda function to convert each Celsius temperature to Fahrenheit using the formula:
- (9/5)×𝑥+32
- list() converts the mapped results to a list.
- print(fahrenheit_temperatures) outputs the Fahrenheit temperatures.
Example 3
# Python example program for map() function
words = ["Welcome","to","Scholarhat"]
# lambda function defines the string operation
concatenated_words = list(map(lambda x : x.capitalize( ) + "!", words))
# print the list of concatenated words
print(concatenated_words)
Output
['Welcome!', 'To!', 'Scholarhat!']
Explanation
- words is a list of strings: ["Welcome", "to", "Scholarhat"].
- map() applies a lambda function to each word:
- x.capitalize() capitalizes the first letter,
- + "!" adds an exclamation mark at the end.
- list() converts the mapped results to a list.
- print(concatenated_words) displays the modified list.
Example 4
Using map () function with tuple
def example(s):
return s.upper()
tuple_exm = ('Welcome','to','Scholarhat')
upd_tup = map(example, tuple_exm)
print(upd_tup)
print(tuple(upd_tup))
Output
<map object at 0x7fd663cb9640>
('WELCOME', 'TO', 'SCHOLARHAT')
Explanation
- example(s) convert a string s to uppercase.
- tuple_exm is a tuple of strings ('Welcome', 'to', 'Scholarhat').
- map(example, tuple_exm) applies the function to each string.
- print(upd_tup) prints the map object (not the values).
- print(tuple(upd_tup)) converts the map object to a tuple and prints it.
Converting Map objects to a list
A map object in Python is an iterator that applies a function to every item of an iterable (like a list) and returns a lazy map object. To access the results, you need to convert it to a list (or another collection type).
def square(x):
return x * x
# Create a list of numbers
numbers = [1, 2, 3, 4, 5]
# Use map to apply the 'square' function to each item
squared_map = map(square, numbers)
# Convert the map object to a list
squared_list = list(squared_map)
# Print the result
print(squared_list)
Output
[1, 4, 9, 16, 25]
Explanation:
- square(x) returns the square of a number.
- numbers = [1, 2, 3, 4, 5] is the list of input numbers.
- map(square, numbers) applies the square function to each number.
- list(...) converts the map object to a list.
- print(squared_list) displays the result.
Using Map in Python with Lambda Functions
The map() function in Python takes in a function and a list as parameters. This function is called with a lambda function and a list, and a new list is returned that contains all the lambda-modified items returned by that function for each item.
Example:
li = [9, 7, 22, 77, 54, 62, 77, 93, 73, 61]
final_list = list(map(lambda x: x*2, li))
print(final_list)
Output
[18, 14, 44, 154, 108, 124, 154, 186, 146, 122]
Explanation:
- A list li is defined with 10 integers.
- map() with lambda x: x*2 doubles each element in the list.
- list() converts the mapped result to a list.
- final_list stores the doubled values.
- print(final_list) displays the new list.
Using Map in Python with Dictionary
Example:
boy_dict ={'a': 'Ankit', 'b': 'vishvaraj', 'c': 'mohan', 'd': 'rocky', 'e': 'Jayesh'}
# adding an '_' to the end of each value
boy_dict = dict(map(lambda x: (x[0], x[1] + '_'), boy_dict.items() ))
print('The modified dictionary is : ')
print(boy_dict)
Output
The modified dictionary is :
{'a': 'Ankit_', 'b': 'vishvaraj_', 'c': 'mohan_', 'd': 'rocky_', 'e': 'Jayesh_'}e
Explanation:
- A dictionary boy_dict is defined with keys 'a' to 'e' and string values (names).
- boy_dict.items() gives key-value pairs as tuples.
- map() with lambda x: (x[0], x[1] + '_') adds an underscore _ to the end of each value.
- dict() converts the modified pairs back into a dictionary.
How to Use Map() With Set
Example
def example(i):
return i%2
set_exm = {24, 102, 62, 46, 44, 28, 222}
upd_itms = map(example, set_exm)
print(upd_itms)
print(set(upd_itms))
Output
{0}
Explanation:
- example(i) returns i % 2 → 0 for even, 1 for odd.
- set_exm contains only even numbers.
- map(example, set_exm) applies the function to each number.
- All results are 0, so set(upd_itms) becomes {0}.
- First print shows a map object, second prints {0}.
String Modification using map()
Example
# List of strings
l = ['mom', 'tom', 'com', 'rom']
# map() can listify the list of strings individually
test = list(map(list, l))
print(test)
Output
[['m', 'o', 'm'], ['t', 'o', 'm'], ['c', 'o', 'm'], ['r', 'o', 'm']]
Explanation:
- l is a list of strings: ['mom', 'tom', 'com', 'rom'].
- map(list, l) converts each string into a list of its characters.
- list(map(...)) turns the result into a list.
- print(test) outputs a list of character lists.
If Statement with map()
In the example, the double_even() function doubles even numbers and leaves odd numbers unchanged. The map() function is used to apply this function to each element of the numbers list, and an if statement is used within the function to perform the necessary conditional logic.Example
# Define a function that doubles even numbers and leaves odd numbers as is
def double_even(num):
if num % 2 == 0:
return num * 2
else:
return num
# Create a list of numbers to apply the function to
numbers = [4, 5, 6, 7, 8]
# Use map to apply the function to each element in the list
result = list(map(double_even, numbers))
# Print the result
print(result)
Output
[8, 5, 12, 7, 16]
Explanation:
- double_even(num) doubles the number if it's even, otherwise returns it unchanged.
- numbers is the list [4, 5, 6, 7, 8].
- map(double_even, numbers) applies the function to each number in the list.
- list(...) converts the result to a list.
- print(result) shows the final list.
Conclusion
In this tutorial, we explored that the Python map() function is a powerful and efficient tool for applying a transformation or function to every item in an iterable. It promotes clean, readable, and concise code by eliminating the need for explicit loops when processing data collections. Throughout this tutorial, we explored the syntax, use cases, and a variety of practical examples using map() with different data structures such as lists, tuples, sets, dictionaries, and strings. To further extend your knowledge in Python, join our free Python programming course.FAQs
Take our Python skill challenge to evaluate yourself!
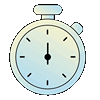
In less than 5 minutes, with our skill challenge, you can identify your knowledge gaps and strengths in a given skill.