25
AprList of Python Keywords (With Examples)
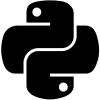
Python Programming For Beginners Free Course
List of Python Keywords: An Overview
Python Keywords are one of the very beginner concepts of the Python language that you definitely need to know for basic Python programs. In this Python Tutorial, you will get to know What is Keywords in Python with examples, Types of Keywords in Python, How to identify Python Keywords and their usage. To delve deeper into various concepts of Python, enroll in our Python Certification Training right now!
Read More: Top 50 Python Interview Questions and Answers
What are Python Keywords?
Keywords in Python are predefined words, that are not to be used as any other kind of identifiers like for example, variable names or function names. These keywords are a part of the syntax in the language with a special meaning associated to them. They play a major role in python code by defining its structure and logic.
Here is a list of keywords in Python:
False | await | else | import | pass |
None | break | except | in | raise |
True | class | finally | is | return |
and | continue | for | lambda | try |
as | def | from | nonlocal | while |
assert | del | global | not | with |
async | elif | if | or | yield |
Python keywords might be different depending on the version you're using, as in, some new ones might get introduced or some existing ones might not be there. So it is better to always check what keywords are there. You can do that with the help of 'keyword' module like this:
import keyword
print(keyword.kwlist)
When you run this code in Python Compiler you will get a list of Python keywords printed as in the below output:
Output
['False', 'None', 'True', 'and', 'as', 'assert', 'async', 'await', 'break', 'class', 'continue', 'def', 'del', 'elif', 'else', 'except', 'finally', 'for', 'from', 'global', 'if', 'import', 'in', 'is', 'lambda', 'nonlocal', 'not', 'or', 'pass', 'raise', 'return', 'try', 'while', 'with', 'yield']
Read More: Python Developer Salary
How to Identify Python Keywords
- Use an IDE With Syntax Highlighting
- Use Code in a REPL to Check Keywords
- Look for a SyntaxError
Classification of Python Keywords
Category | Description | Example |
Reserved | The reserved keywords are the ones that are reserved for specific purposes. | if, else, for, while, return, etc. |
Control Flow | The control flow keywords control the execution flow in Python programs. | if, else, elif, for, while, break, continue, return, etc. |
Declaration | The declaration keywords are used for declaring variables, functions, classes and other entities. | def, class, lambda, global, nonlocal, etc. |
Exception Handling | The exception handling keywords are used for exception handling purposes. | try, except, finally, raise, assert, etc. |
Miscellaneous | The miscellaneous keywords have their own unique purposes in python. | True, False, None, and, or, not, etc. |
Read More: Python Career Opportunities: Is it worth learning Python in 2024?
Python Keywords and Their Usage
1. Value Keywords
- True, False- They both are used in logical expressions and represent the boolean values 'True' and ' False'.
- None- It is used to represent that there is no value or it is null.
Example of Value keywords
print(True)
print(False)
print(None)
Output
True
False
None
2. Operator Keywords
- and-It will return 'True' when both the operands are 'True'.
- or-It will return 'True' when at least one of the operand is 'True'.
- not-It will return the opposite of the operand.
- in-It checks that whether a specified value is present in the given sequence.
- is-It compares the identity of objects.
Example of Operator keywords
a = True
b = False
print(a and b)
print(a or b)
print(not a)
print(5 in [1, 2, 3, 4, 5])
print(5 is 5)
Output
False
True
False
True
True
3. Control Flow Keywords
- if-It will execute a code block if the condition is 'True'.
- elif-It is the short form of 'else if'. It will put another alternative condition for checking if the 'if' condition is 'False'.
- else-It will execute a code block if none of the conditions are 'True'.
Example of Control Flow keywords
x = 10
if x > 5:
print("x is greater than 5")
elif x == 5:
print("x is equal to 5")
else:
print("x is less than 5")
Output
x is greater than 5
4. Iteration Keywords
- for-It iterates over the elements of a specified sequence.
- while-It will execute a code block as long as the condition is 'True'.
- break-It is used for terminating the loop before completion.
- continue-It is used for skipping the current iteration and continue with the next one.
- else-It will execute a code block when the has completed without a 'break' statement.
Example of Iteration keywords
for i in range(5):
if i == 3:
break
print(i)
else:
print("Loop completed successfully")
Output
0
1
2
5. Structure Keywords
- def-It defines a function.
- class-It defines a class.
- with-It uses context managers to simplify resource management.
- as-It renames or aliases imported modules or objects.
- pass-It indicates that no action needs to be taken.
- lambda- It creates an anonymous function.
Example of Structure keywords
def example_function():
pass
class ExampleClass:
pass
with open('example.txt', 'r') as file:
data = file.read()
lambda_func = lambda x: x * x
print(lambda_func(5))
Output
25
6. Returning Keywords
- return-It will exit a function and may also return a value.
- yield-It will stop the execution of a function and will return a value to the caller while the state of the function remains constant.
Read More: Functions in Python
Example of Returning keywords
def example_function():
return 42
def generator_function():
yield 1
yield 2
yield 3
print(example_function())
print(list(generator_function()))
Output
42
[1, 2, 3]
7. Import Keywords
- import-It will import a whole module.
- from-It will import specific symbols from a module.
- as-It will alias the imported symbols or modules.
Example of Import keywords
import math
from datetime import datetime as dt
print(math.pi)
print(dt.now())
Output
3.141592653589793
<current date and time>
8. Exception-Handling Keywords
- try-It will start and check a code block that may have an exception but not execute it.
- except-It is used for checking and handling exceptions.
- raise- It will raise an exception.
- finally-It will execute a code no matter if any exceptions occurs or not.
- else-It will execute the code if there is no exception occurring in the 'try' block.
- assert-When the condition is 'False', an 'AssertionError' is raised to assert a condition.
Example of Exception-Handling keywords
try:
x = 1 / 0
except ZeroDivisionError:
print("Error: Division by zero")
else:
print("No error occurred")
finally:
print("Cleanup code")
assert 2 + 2 == 4, "Math is broken"
Output
Error: Division by zero
Cleanup code
9. Asynchronous Programming Keywords
- async-It is used for defining a coroutine function.
- await-It will pause the execution of a coroutine function until its own coroutine function completes.
Example of Asynchronous Programming keywords
import asyncio
async def example_coroutine():
await asyncio.sleep(1)
print("Async function executed")
async def main():
await example_coroutine()
asyncio.run(main())
Output
Async function executed
10. Variable Handling Keywords
- del-It will delete a variable from a collection.
- global-It is used for declaring a variable with a global scope.
- nonlocal-It is used for declaring a variable with a scope of the nearest function just outside the current one.
Example of Variable Handling keywords
x = 10
print(x)
del x
print(x) # Raises NameError because x is deleted
Output
10
NameError: name 'x' is not defined