25
AprBoolean and Static in C Programming With Examples ( Full Guide )
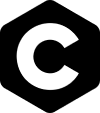
C Programming For Beginners Free Course
Boolean and Static in C: An Overview
Are you a beginner who wants to learn the basics of the C programming language? Our C for Beginners course is designed just for you. In this comprehensive program, we will explain the inherent components of C and other related languages. We will discuss their applications, cover use cases for each concept, and present illustrated diagrams to aid your comprehension. Join our C online training and gain a solid foundation in programming with practical insights and hands-on learning.
Boolean in C
The bool data type is one of the fundamental data types in C. It is used to hold only two values i.e. true
or false
. The C program returns boolean values as integers, 0
and 1
.
The boolean meaning of these integers are:
0
indicates afalse
value1
(or any other number not equal to 0) indicatestrue
value.
As they return boolean values, we need to use the format specifier, “%d
” to print the boolean values.
Syntax
In C language, the bool data type is not a built-in data type. The C99 standard for C language introduced boolean in C. It is necessary to import the header file,stdbool.h
to declare boolean variables to use boolean value in C.bool variable_name;
The “bool
” keyword is used to declare boolean variables.
Example
#include <stdio.h>
#include <stdbool.h>
int main()
{
bool x=false, y=true; // variable initialization.
printf("%d\n",x);
printf("%d\n",y);
return 0;
}
In the above code in the C Compiler, the boolean variables, x
, and y
are initialized with the value, “false
” and “true
” respectively. The code prints the boolean values as integers.
Output
0
1
Read More - Top 50 Mostly Asked C Interview Questions
How to use a boolean in C?
- Boolean variables: the user can declare Boolean variables to store Boolean values.
For example:
#include <stdbool.h> bool isSunny = true; bool isRaining = false;
- Boolean expressions: the user can use Boolean expressions to evaluate conditions and make decisions in their code.
For example:
if (isSunny && !isRaining) { printf("It's a perfect day for a picnic!\n"); }
The above code uses an
if
statement to check the condition. The test expression uses the logical operator,&&
to evaluate the condition. If the test expression results in the boolean value, “true
”; theif
statement will be executed. The use of booleans in decision-making and looping statements will be seen in the upcoming sections. - Boolean functions: The developer can write boolean functions that return true or false depending on some condition.
For example:
bool isEven(int num) { return (num % 2 == 0); }
In the above code, the function
isEven()
returns a boolean value in C. You will get more clarity on functions in the section, Functions in C. - Boolean arrays: the user can use boolean arrays to store a collection of boolean values in C.
For example:
bool weekdays[7] = { true, true, true, true, true, false, false };
The above code declares an
array
of size7
. The array stores 7 boolean values, “true
” and “false
”. We use thefor
loop and indexing to access these values. When we will study arrays in the section Arrays in C, you will get more ideas regarding this example.
What is Static in C?
Static in C is a keyword to indicate a particular memory location that remains occupied during the program’s lifetime. In essence, defining something as static
makes it available throughout the entire program, which is particularly useful when wanting to keep values persistent between function calls.
Static variables enable data to remain after the function has been executed and returned; this allows the function to remember a value from a previous call, making them both efficient and powerful.
How to use static keywords in C?
The static
keyword can be used with both variables and functions.
- Static variable
Syntax
static data_type variable_name;
- Static Global Variable
When a C language’s global variable is declared with a static
keyword, it is known as a static global variable. It is declared at the top of the program, and its visibility is throughout the program
Example
#include <stdio.h>
static char c;
static int i;
static float f;
static char s[100];
int main ()
{
printf("%c %d %f %s",c,i,f,s); // the default values of c, i, f, and s will be printed.
return 0;
}
c
' (char), 'i
' (int), 'f
' (float), and 's
' (char array) are declared with global scope. So, it publishes the initial default values for the letters "c
," "i
," "f
," and "s
," which are 0
, 0
, 0.0
, and an empty string
respectively.
Output
0 0 0.000000 (null)
- Static Local Variable
It is a local variable, declared with a static
keyword. The memory of a static local variable is valid throughout the program, but the scope of visibility of a variable is the same as the automatic local variables. However, when the function modifies the static local variable during the first function call, this modified value will be available for the next function call also.
Example
#include <stdio.h>
int main()
{ printf("%d",function());
printf("\n%d",function());
return 0;
}
int function()
{ static int count=0;
count++;
return count;
}
In the above code, the count
variable is a static
variable. When the function, “function()”
is called first time, the value of count
increments to 1, and during the next function call, the value of the count
variable becomes 2.
Output
1
2
- Static member variables
When the member variables are declared with a static
keyword in a class, then it is known as static
member variables. They can be accessed by all the instances of a class, not with a specific instance.
- Static Function
It is a function declared with a static
keyword. Its lifetime is throughout the program. The static
function can be accessed within a file only.
Example
#include<stdio.h>
static void function()
{
printf("Welcome to the ScholarHat");
}
int main() {
function();
return 0;
}
The above code in the C Editor creates a static function named function()
. This function()
is called by the main function.
Output
Welcome to the ScholarHat
- The Static Method
The member function of a class declared with a static keyword is known as a static method. It is accessible to all the instances of a class and not only to a specific instance.
Summary
Boolean and Static in C are two key concepts in a programming language. Boolean data type assigns either true or false values to any statement or expression. On the other hand,static
is a keyword that allows only one copy of a variable to be shared between multiple functions. Consider enhancing your knowledge and gaining recognition by enrolling in C Certification Training, which will provide comprehensive guidance and hands-on experience in mastering various concepts and techniques in C programming.