25
AprConditional Statements in C: if, if..else, Nested if
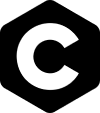
C Programming For Beginners Free Course
Conditional Statements in C: An Overview
If you are a programmer, then you already know how to use if...else
statements. If not, it's time to learn! Understanding if...else
statements is essential, especially for beginners. After reading this article in the C tutorial, you will have a fundamental understanding regarding the use of if...else
statements in C programming. However, if you're looking to solidify your knowledge and demonstrate your proficiency in C programming, consider enrolling in our C certification course.
What are Conditional Statements in C?
The conditional statements are also known as decision-making statements. They are of the type if statement
, if-else
, if else-if ladder
, switch
, etc. These statements determine the flow of the program execution.
Why Conditional Statements?
- Dynamically controls the manner of program execution.
- Useful in error and exception handling.
- Check the flow of your program.
- Helps to manage the state of your program.
- Helpful in performing complex calculations.
Types of Conditional Statements in C
In this article, we'll discuss the first kind of conditional statement i.e. if...else
statements in C. We'll discuss the switch
statement in the next tutorial switch Statement in C.
1. if … else statements in C
if-else
is the first kind of control statement in C. In this, the operations are performed according to some specified condition. The statements inside the body of the if
block get executed if and only if the given condition is true.
Read More - Top 50 C Interview Questions and Answers
Variants of if-else statements in C
We have four variants ofif-else
statements in C. They are
if
statement in Cif-else
statement in Cif else-if ladder
in C-
nested if
statement in C
- if statement in C
When you want to print any code only upon the satisfaction of a given condition, go with the simple if
statement.
Flow Chart:
Syntax
if(test condition){
//code to be executed
}
Example
#include<stdio.h>
int main(){
int num=8;
if(num%2==0){
printf("%d is even number",num);
}
return 0;
}
- The above code in the C Compiler prints the even number.
- The
if
statement checks if the user input is odd or even. - If the number is even, the statement inside the
if
block gets printed. - If the given input is odd, nothing gets printed.
Output
8 is even number
- if-else statement in C
It is an extension of the if
statement. Here, there is an if
block and an else
block. When one wants to print output for both cases - true
and false
, use the if-else
statement.
Syntax
if(test condition){
//code to be executed if condition is true
}else{
//code to be executed if condition is false
}
Example
#include <stdio.h>
int main() {
int num = 10;
if(num < 5) {
printf("Number is less than 5\n");
}
else if(num < 10) {
printf("Number is between 5 and 9\n");
}
else {
printf("Number is 10 or greater\n");
}
return 0;
}
- In the above code, the
if
condition checks if thenum
is less than 5 - The given number 10 is greater than 5.
- Therefore, the
if
block gets skipped. - The statement inside the
else
block gets printed.
Output
Number is 10 or greater
- if else-if ladder in C
It is an extension of the if-else
statement. If we want to check multiple conditions if the first “if
” condition becomes false, use the if else-if ladder
. If all the if
conditions become false the else
block gets executed.
Flow Chart:
Syntax
if(test condition1){
//code to be executed if condition1 is true
}else if(test condition2){
//code to be executed if condition2 is true
}
else if(test condition3){
//code to be executed if condition3 is true
}
...
else{
//code to be executed if all the conditions are false
}
Example
#include <stdio.h>
int main(){
int number=5;
if(number==20){
printf("number is equals to 20");
}
else if(number==50){
printf("number is equal to 50");
}
else if(number==100){
printf("number is equal to 100");
}
else{
printf("number is not equal to 20, 50 or 100");
}
return 0;
}
- In the given code, the program evaluates multiple conditions.
- If the first
if
condition becomes false, the control goes to theelse-if
statements. - If all the conditions fail, the statement inside the
else
block gets printed.
Output
number is not equal to 20, 50 or 100
- Nested if…else statement in C
We can include an if-else
block in the body of another if-else
block. This becomes a nested if-else
statement.
Syntax
if(test condition1)
{ //if condition1 becomes true
if(test condition1.1)
{ //code executes if both condition1 and condition 1.1 becomes true
}
else
{ //code executes if condition1 is true but condition 1.1 is false
}
}
else
{ //code executes if condition1 becomes false
}
Example
#include <stdio.h>
int main()
{
int a = 10;
int b = 5;
if (a > b)
{
// control goes to the nested if
if (a % 2 == 0) {
printf("%d is even\n",a);
}
else {
printf("%d is odd\n",a);
}
}
else
{
printf("%d is not greater than %d\n",a,b);
}
return 0;
}
- In the above code in the C Online Compiler, the first
if
statement checks whethera>b
. - If
a
is greater thanb
, thenested if
statement is checked. - If the
nested if
condition isfalse
, theelse
statement in thenested if
block gets executed.
If the first
if
statement evaluates to false
, the nested
if
block gets skipped and the else
block executes.Output
10 is even
2. Switch Statement in C
A switch statement tests a variable for equality against a list of values. Each value is called a case, and the variable being switched on is checked for each switch case.
Flowchart:
Syntax of Switch Statement in C
switch(expression){
case value1:
//code to be executed;
break; //optional
case value2:
//code to be executed;
break; //optional
......
default:
code to be executed if all cases are not matched;
}
Example of Switch Statement in C
#include <stdio.h>
int main()
{
int var = 3;
switch (var) {
case 1:
printf("Case 1 is executed");
break;
case 2:
printf("Case 2 is executed");
break;
case 3:
printf("Case 3 is executed");
break;
default:
printf("Default Case is executed");
break;
}
return 0;
}
Output
Case 3 is executed
Read More: Switch Statement in C: Syntax and Examples
3. Conditional Operator in C
The ? is called a ternary operator/conditional operator because it requires three operands and can be used to replace if-else statements.
Syntax of Conditional Operator in C
testexpression? expression1 : expression2;
Example of Conditional Operator in C
#include <stdio.h>
int main() {
int a = -4;
double b = 29.42;
int c = a? printf("True value : %lf",b):printf("False value : 0");
return 0;
}
Output
True value: 29.420000
Read More: Ternary Operator in C: Ternary Operator vs. if...else Statement
4. Jump Statements in C
C language provides the flexibility of jumping from one statement to another. There are four kinds of jump statements in C:
1. break
In C, break is used to prematurely exit from a loop or switch statement, before the block has been fully executed.
Flowchart
Syntax of break statement in C
//loop or switch case
break;
Example of break statement in C Playground
#include <stdio.h>
main( ){
int i;
for (i=1; i<=5; i++){
printf ("%d ", i );
if (i==5)
break;
}
}
Output
1 2 3 4 5
2. continue
In contrast to the break statement, instead of terminating the loop, continue forces to execute the next iteration of the loop.
Syntax of continue statement in C
//loop statements
continue;
//some lines of the code which is to be skipped
Example of continue statement in C
#include <stdio.h>
int main( ){
int i;
for (i=1; i<=8; i++){
if (i==5)
continue;
printf("%d ", i);
}
return 0;
}
Output
1 2 3 4 6 7 8
Read more about break and continue statements in Continue Statement in C: What is Break & Continue Statement in C with Example
3. goto
It is used after the normal sequence of program execution by transferring the control to some other part of the program.
Syntax of goto statement in C
goto label;
// ...
label:
// Statement(s) to execute
Example of goto statement in C
#include <stdio.h>
int main( ) {
printf("Welcome ");
goto l1;
printf("How's the experience?");
l1: printf("to ScholarHat");
return 0;
}
Output
Welcome to ScholarHat
4. return
It terminates the execution of the function and returns the control of the calling function.
Syntax of return statement in C
return expression;
Example of return statement in C
#include <stdio.h>
int multiply(int x, int y) {
return x * y;
}
int main() {
int z = multiply(8,9);
printf("The product is: %d\n", z);
return 0;
}
Output
The product is: 72
Read more about goto and return statements in Jump Statements in C: break, continue, goto, return.
Summary
if...else
statements in C are a crucial part of writing an efficient program. They help to organize code and can account for a variety of conditions using comparison operators and logical operators. If used correctly, if...else
statements can save your time from writing multiple lines of code. Knowing how to use them properly is thus essential for any programmer. You can also check out our C Certification Training to test your knowledge and get comfortable with using if...else
statements.