14
JunData Types in C Programming - A Beginner Guide with examples
Data Types in C
Data Types in C are the data values a C program can process. They are the basic building blocks of a C program.If you want to get an idea of Data Types in C, Think of it this way: just like you sort your wardrobe into shirts, pants, and accessories, C organizes and handles data by defining different types like integers, characters, and floating-point numbers.It is something we already saw in the variables in C and keywords in the C article.
In this C Tutorial for Beginners,We are going to look at the wide variety of data types in Cas well as their importance and applications. To gain a deeper understanding, you can enroll in a C programming language online course free and start learning today!
What is DataTypes?
Data types in C refer to the various types of data, such as integer and float, that a C program can process. Each type of data is represented by a specific keyword and is used within a program to perform calculations, represent objects, or store strings.
- A data type defines how a certain operation will be performed on the data item specified as its arguments such as an arithmetic operation or a comparison test.
- Data types also determine the amount of memory allocated for storing a particular type of value.
- Data types are essential to C programming since they are necessary to define the items used within any program.
Read More - Top 50 Mostly Asked C Interview Questions and Answers
Types of Data Types in C with Examples
There are various types of data types in C, which are:
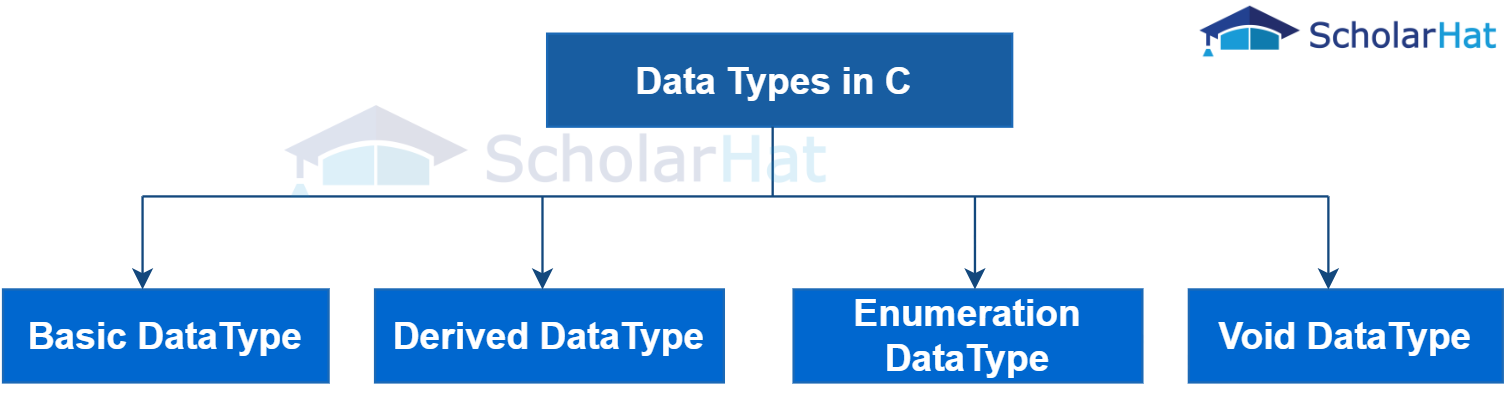
Types | Data Types |
Basic Data Type | int, char, float, double |
Derived Data Type | array, pointer, structure, union |
Enumeration Data Type | enum |
Void Data Type | void |
1. Basic or Primitive Data Types in C
The fundamental data types in C, such as integers, float, characters, etc., are used to represent simple values and are known as the "basic data types."
1. int
The int data type is used to store integers (whole numbers) like -1, 0, 42, etc.
Syntax
int variable_name;
Range of Values of int Data Type in C
Data Type | Format Specifier | Range | Memory Size (in bytes) |
Int | %d | -2,147,483,648 to 2,147,483,647 | 4 |
short int | %hd | -32,768 to 32,767 | 2 |
unsigned int | %u | 0 to 4,294,967,295 | 4 |
unsigned short int | %hu | 0 to 65,535 | 2 |
long int | %ld | -2,147,483,648 to 2,147,483,647 | 4 |
unsigned long int | %lu | 0 to 4,294,967,295 | 4 |
long long int | %lld | -(2^63) to (2^63)-1 | 8 |
unsigned long long int | %llu | 0 to 18,446,744,073,709,551,615 | 8 |
Example of int Data Type in C Compiler
#include <stdio.h>
int main()
{
// Integer value with positive data.
int a = 9;
// integer value with negative data.
int b = -9;
// U or u is Used for Unsigned int in C.
int c = 89U;
// L or l is used for long int in C.
long int d = 99998L;
printf("Integer value with positive data: %d\n", a);
printf("Integer value with negative data: %d\n", b);
printf("Integer value with an unsigned int data: %u\n",
c);
printf("Integer value with an long int data: %ld", d);
return 0;
}
Output
Integer value with positive data: 20
Integer value with negative data: -20
Integer value with an unsigned int data: 89
Integer value with an long int data: 99998
Common Usage Scenarios of int Data Type in C
- Counting: Integers are commonly used for counting discrete objects or events. For example, counting the number of students in a class, the number of days in a week, etc.
- Indexing: Integers are often used as indices to access elements in arrays, lists, or other data structures.
- Mathematical Operations: Integers are used in mathematical calculations such as addition, subtraction, multiplication, and division.
- Loop Iterations: Integers are frequently used as loop counters in iteration constructs like for loops and while loops.
- Memory Allocation: Integers are used to represent memory addresses, offsets, and sizes.
- Error Codes and Flags: Integers are often used to represent error codes, status flags, and boolean values.
- User Input and Output: Integers are commonly used to represent user input from keyboards, mouse clicks, or other input devices.
2. char
The char data type is used to store single characters like 'A', '1', or '$'. The character must be surrounded by single quotes and we must use the %c format specifier to print it.
Syntax
char variable_name;
Example of char Data Type in C
#include <stdio.h>
int main() {
char myLetter = 'S';
printf("%c", myLetter);
return 0;
}
Output
S
Representing single characters using ASCII values
You can also use ASCII values to display certain characters. Here, these values are not surrounded by quotes (' '), as they are numbers
Example
#include <stdio.h>
int main() {
char a = 87, b = 66, c = 75;
printf("%c\n", a);
printf("%c\n", b);
printf("%c", c);
return 0;
}
Output
W
B
K
3. float
The float data type is used to store single-precision floating-point numbers, which can represent decimal values with limited precision. This data type size is 4 bytes, i.e., it occupies 4 bytes of memory. It ranges between +/- 3.4E-38F up until +/- 1 .7E+308F depending on the system’s architecture.
Syntax
float variable_name;
Example of float Data Type in C
#include <stdio.h>
int main() {
float myNum = 6.85;
printf("%f", myNum);
return 0;
}
Output
6.850000
4. double
The double data type is used to store double-precision floating-point numbers i.e. 64 bits of decimal numbers or floating points, which offer higher precision than float for decimal values. This data type size is 8 bytes, i.e., it occupies 8 bytes of memory. It ranges between 1.7E-308 to 1.7E+308.
Syntax
double variable_name;
Example of double Data Type in C Online Compiler
#include <stdio.h>
int main() {
double num = 20.63;
printf("%lf", num);
return 0;
}
Output
20.630000
float vs. double
- The precision of a floating point value indicates how many digits the value can have after the decimal point.
- The precision of float is six or seven decimal digits, while double variables have a precision of about 15 digits.
- Therefore, it is recommended to use double for most calculations. However, double takes up twice as much memory as float (8 bytes vs. 4 bytes).
How to set Decimal Precision in C?
If you want to remove the extra zeros from a floating point number or in other words, set decimal precision, you can use a dot (.) followed by a number that specifies how many digits should be shown after the decimal point.
Example
#include <stdio.h>
int main() {
float floatNum = 7.5;
printf("%f\n", myFloatNum); // Default will show 6 digits after the decimal point
printf("%.1f\n", myFloatNum); // shows 1 digit
printf("%.2f\n", myFloatNum); // shows 2 digits
printf("%.4f", myFloatNum); // shows 4 digits
return 0;
}
Output
7.500000
7.5
7.50
7.5000
5. void
When a function doesn't return a value or a pointer doesn't have a particular data type attached to it, C uses the void data type to express this. It is commonly used in function declarations as a return type indicating that the function does not return any values and that it simply performs some task without producing any results.
Syntax
void functionName(parameters) {
// Function code
}
#include <stdio.h>
int main()
{
int val = 50;
void* ptr = &val;
printf("%d", *(int*)ptr);
return 0;
}
Output
50
2. Derived Data Types in C
In the C programming language, a derived data type is a data type that is created by combining one or more basic or other derived data types.
1. Arrays
An array is a group of identically typed elements kept in consecutive memory regions. Multiple values of the same type can be stored and accessed using a single variable name.
Syntax
data_type array_name[array_size];
Initializing Array in C
There are various ways to do this:- Initialize at the time of declaration using the “
{}
”.int a[5] = {1, 2, 3, 4, 5};
- Initialize an array without specifying its size at declaration time.
int a[] = {1, 2, 3, 4, 5};
Though we haven't specified the size, the compiler understands the size as 5 due to the initialization of 5 elements.
- Initialization by using the index of an element
int marks[5]; marks[0]=80; //the index of an array starts with 0. marks[1]=60; marks[2]=70; marks[3]=85; marks[4]=75;
There are two types of array
in C, which are:
- Single-dimensional array: It is a collection of elements of the same data type that are stored in a contiguous block of memory.
- Multi-dimensional array: It is an array that contains one or more arrays as its elements. We will see this in the next section multidimensional arrays in C.
For more details on arrays: Arrays in C Programming
2. Pointers
A pointer is a variable that keeps track of another variable's memory address. Pointers are used to indirectly access data by referencing its location in memory and for dynamic memory allocation.
Syntax
data_type *pointer_name;
Example of Pointers in C
#include <stdio.h>
int main(){
int number=120;
int *p;
p=&number;//stores the address of number variable
printf("Address of p variable is %x \n",p); // p contains the address of the number therefore printing p gives the address of number.
printf("Value of p variable is %d \n",*p); // As we know that * is used to dereference a pointer therefore if we print *p, we will get the value stored at the address contained by p.
return 0;
}
Output
Address of number variable is fff4
Value of p variable is 120
Pointer Arithmetics in C
- Increment/Decrement of a Pointer
Example of Increment/Decrement Pointers in C
#include <stdio.h> int main() { int a = 22; int *p = &a; printf("p = %u\n", p); // p p++; printf("p++ = %u\n", p); //p++ = p +4 // 4 bytes p--; printf("p-- = %u\n", p); //p-- = p -4 // restored to original value return 0; }
Output
p = 1357824204 p++ = 1357824208 p-- = 1357824204
- Addition of Integer to Pointer
Example of Addition of Integer to Pointer in C
#include <stdio.h> int main() { int a = 10; int *ptr1, *ptr2; ptr1 = &a; ptr2 = &a; printf("Pointer ptr2 before Addition: "); printf("%p \n", ptr2); ptr2 = ptr2 + 3; printf("Pointer ptr2 after Addition: "); printf("%p \n", ptr2); return 0; }
Output
Pointer ptr2 before Addition: 0x7ffea5bf8804 Pointer ptr2 after Addition: 0x7ffea5bf8810
- Subtraction of Integer to Pointer
Example of Subtraction of Integer to Pointer in C Editor
#include <stdio.h> int main() { // Integer variable int N = 10; int *ptr1, *ptr2; ptr1 = &N; ptr2 = &N; printf("Pointer ptr2 before Subtraction: "); printf("%p \n", ptr2); ptr2 = ptr2 - 3; printf("Pointer ptr2 after Subtraction: "); printf("%p \n", ptr2); return 0; }
Output
Pointer ptr2 before Subtraction: 0x7ffe0a32d8e4 Pointer ptr2 after Subtraction: 0x7ffe0a32d8d8
Read More: Pointers in C
3. User-Defined Data Types in C
1. Structure
A user-defined composite data type called a "structure" brings together variables of several data kinds under one name. Complex data structures are made using it to represent real-world entities. You can create a structure by using the struct keyword and declare each of its members inside curly braces
Syntax
struct structure_name {
data_type member1;
data_type member2;
// Add more members as needed
};
Access Structure Members
To access the structure, create a variable of it. Use the struct keyword inside the main() method, followed by the name of the structure and then the name of the structure variable. To access members of a structure, use the dot syntax (.) after the structure variable.
#include <stdio.h>
// Create a structure called myStructure
struct scholarHat {
int myNum;
char myLetter;
};
int main() {
// Create a structure variable of scholarHat called s
struct scholarHat s;
// Assign values to members of s
s.myNum = 15;
s.myLetter = 'S';
// Print values
printf("My number: %d\n", s.myNum);
printf("My letter: %c\n", s.myLetter);
return 0;
}
Output
My number: 15
My letter: S
2. Union
The union data type in C is a user-defined datatype that allows users to store different data types in the exact memory location. It is similar to a structure but with one important difference, i.e., only one of its members can be active and contain valid information at any given point in time.
Syntax
union union_name {
data_type member1;
data_type member2;
// Add more members as needed
};
#include <stdio.h>
union Data
{ int x;
float y;
}
data;
int main()
{
data.x = 35;
printf("data.x: %d\n", data.x);
data.y = 6.5f ;
printf("data.y: %f", data.y);
return 0;
}
Output
data.x: 35
data.y: 6.500000
3. Enumeration
A user-defined data type called enumeration in C consists of a finite collection of named integer constants that are often used to represent a group of related values with unique names. To create an enum, use the enum keyword, followed by the name of the enum, and separate the enum items with a comma. To access the enum, you must create a variable of it.
Syntax
enum enum_name {
constant1,
constant2,
constant3,
// Add more constants as needed
};
#include <stdio.h>
enum ScholarHat {
LOW,
MEDIUM,
HIGH
};
int main() {
// Create an enum variable and assign a value to it
enum ScholarHat var = MEDIUM;
// Print the enum variable
printf("%d", var);
return 0;
}
Output
1
Datatypes in a Nutshell
Type | Description | Examples |
Primitive Data Types | The most basic data types built into the C language, are used for representing simple values such as numbers, characters, etc. These data types determine the type and size of data a variable can hold. |
|
Derived Data Types | Data types that are derived from primitive types, allow more complex data structures and functionalities. |
|
User-Defined Data Types | Custom data types are created by the user to represent more complex entities or group-related data under a single type. |
|
Why do we need data types in C?
- Memory Allocation: Data types determine the amount of memory allocated to a variable. Different data types occupy different amounts of memory, allowing efficient use of resources.
- Data Representation: Data types define how data is represented in binary form, ensuring that the computer understands how to manipulate and store values.
- Operations and Functions: Data types determine which operations and functions are valid for a variable. For example, arithmetic operations vary depending on data types (e.g., integer vs. floating-point).
- Compatibility: Data types ensure compatibility when sharing data between different parts of a program or when interfacing with external libraries or hardware.
- Optimization: Properly choosing data types can lead to optimized code. For example, using smaller data types for variables that don't require large ranges can save memory and improve performance.
- Code Readability: Data types make code more readable and self-documenting. They convey the intended use of a variable to other developers.
- Portability: Data types help ensure code portability across different platforms and compilers by defining the size and behavior of variables consistently.
- Memory Management: Data types influence how memory is managed and released, which is crucial for preventing memory leaks and efficiently using system resources.
Summary
In Conclusion, We have explored a vast overview of what is data type in C language including its various types of data types in C with examples. In this article, we have also covered the size of data types in C. If you want to further understand the loop and its application in various C programs, you can consider our C Certification Course.