Top 30 React Hooks Interview Questions & Answers
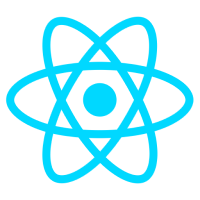
ReactJS Course
React Hooks Interview Questions & Answers: An Overview
In this article, we will explore React Hooks Interview Questions and Answers for beginners, and React Hooks Interview Questions and Answers for experienced professionals. Additionally, we'll also delve into React Certification Training and provide a comprehensive React Tutorial to help you enhance your Data Scientist skills.React Hooks Interview Questions and Answers for Fresher
1. What are React Hooks?
React Hooks are a new feature in React that allows you to use state as well as other React features without having to write a class. They are a more logical and efficient method of writing React components.
2. What are the benefits of using React Hooks?
React Hooks have the following benefits:
- Reuse States & Components.
- Better Code Composition.
- Performance.
- Better Testing.
3. What is the difference between a class component and a functional component with hooks?
- The conventional method for writing React components is using class components.
- They manage state and lifecycle methods with classes.
- React has more recently added functional components.
- To control state and lifecycle methods, they use functions.
- Functional components include a feature called React Hooks that lets them use state as well as additional React capabilities without having to write a class.
4. Explain the difference between state and props in React.
A component's state is data that it owns and manages. It can be updated over time, and modifications will cause the component to re-render. Props are pieces of information that are transferred from a parent component to a child component. They are read-only, and the child component cannot change them.
Read More - React developer salary in India
5. What are the rules of Hooks in React?
Hooks in React follow two key rules:
- Hooks should only be called at the top level of your React function.
- Hooks should only call from React Function not be called within loops, conditions, or nested functions.
6. How do you manage side effects in React functional components with hooks?
The use Effect hook is used in React functional components to manage side effects. Data fetching, subscriptions, and manually modifying the DOM are examples of side effects that might influence things outside of the render function.
7. What is the useState hook and how is it used?
The useState hook is used in React functional components to manage state. It lets you generate and read a state variable, as well as update the state variable.
8. What are the different ways to update the state in React?
In React, there are two primary methods for updating state:
- Using a class component's setState function.
- Using a functional component's useState hook.
9. How do you share the state between components in React?
In React, there are many ways to transfer state between components:
- Props are passed from parent to child components.
- Making use of a global state management library such as Redux or MobX.
- Making use of the Context API.
Read More - Top 50 Mostly Asked React Interview Question & Answers
10. How do you create custom hooks in React?
Custom hooks allow you to reuse stateful functionality across components. They are nothing more than functions that return other Hooks.
React Hooks Interview Questions and Answers for Intermediate
1. What is the useMemo hook and how is it used?
The useMemo hook works to memoize functions within React. Memoization is a technique for caching the result of a function call so that it does not have to be recalculated every time the function is used. This can increase the performance of your React applications.
2. What is the useEffect hook and how is it different from the useLayoutEffect hook?
The useEffect hook is used in React functional components to manage side effects. The useLayoutEffect hook is a subhook of the useEffect hook that is used for side effects that must be performed before the browser paints the next frame.
3. How do you memoize functions in React?
In React, there are two primary methods for memoizing functions:
- The useMemo hook is being used.
- Using a third-party library.
4. How do you handle errors in React functional components with hooks?
With hooks, there are numerous ways to handle problems in React functional components:
- The try/catch block is used.
- Making use of an error boundary component.
- Making use of a third-party library, such as react-error-boundary.
5. What is the useContext hook and how is it used?
The useContext hook is used to access and modify a React component's context value. Context allows components to share data without having to pass props down the component tree.
6. How do you use the useReducer hook for complex state management?
The useReducer hook in React is an effective tool for managing complex states in a more organized as well as predictable manner. It's especially handy when dealing with states with several sub-values or when the future state is dependent on the prior one.
7. How do you fetch data from an API in React with hooks?
Hooks can be used to retrieve data from an API in many ways in React:
- Making use of the fetch API.
- Using an HTTP client library like Axios or SWR.
8. What is the useSWR hook and how is it used?
The useSWR hook is a React hook that provides a solution for data fetching and caching in React apps. It is a wrapper for the axios library that adds capabilities like caching and automatic revalidation.
9. How do you handle data caching and invalidation in React with hooks?
Caching and invalidation of data are critical for improving the speed of React apps. Caching data can increase application performance by reducing the number of times data must be fetched from the server. The practice of ensuring that cached data is up to date is known as invalidation.
With hooks, there are numerous ways to handle data caching and invalidation in React:
- Caching functions with the useMemo hook.
- Invalidating cached data with the useEffect hook.
- Making use of a third-party library like react-query or useSWR.
10. What is the useRef hook and how is it used?
The useRef hook creates and manages mutable references to values. This can be useful for storing non-reactive data, such as a DOM element or a subscription.
React Hooks Interview Questions and Answers for Experienced
1. What is the useTransition hook and how is it used?
The useTransition hook works for coordinating multiple state updates along with transitions. It gives you the ability to modify the timing & animation of state updates.
2. What is the useFetcher hook and how is it used?
The useFetcher hook is used to retrieve information from an API. It is a more powerful and adaptable substitute for the useSWR hook.
3. What is the useSubscription hook and how is it used?
The useSubscription hook works to subscribe to a data stream. It is utilized in real-time applications such as chat applications.
4. How do you test React functional components with hooks?
Hooks can be used to test React functional components in multiple ways:
- Make use of a testing library, such as Jest or the React Testing Library.
- Use a snapshot testing library, such as Jest Snapshot or Storybook, to test your code.
5. What are some of the best practices for using React hooks?
Here are some best practices for using React hooks:
- Hooks can be used to control state and side effects.
- To reuse stateful logic, utilize custom hooks.
- To remember functions, use the useMemo hook.
- To manage side effects, utilize the useEffect hook.
- Use the useReducer hook to manage complex states.
- For data retrieval and caching, utilize a third-party library such as react-query or useSWR.
6. What are some of the common mistakes to avoid when using React hooks?
Here are some common errors to avoid while utilizing React hooks:
- Hooks can be called within loops, conditions, or nested functions.
- Directly changing the condition.
- Using an excessive number of hooks in a single component.
- Custom hooks are not used to reuse stateful logic.
7. What are some of the limitations of React hooks?
Some of the limitations of React hooks are as follows:
- They may be more difficult to understand than class components.
- They can make debugging components more complex.
- In some circumstances, they may be less performant than class components.
8. What are some of the alternative state management libraries for React?
Here are some other React state management libraries:- Redux
- Hookstate
- Jotai
- Rematch
- MobX
- Recoil
- Zustand
9. Can a custom react hook return JSX
No, JSX should not be returned by a custom React hook. Custom React hooks are typically used to encapsulate and reuse stateful behavior across components. They are functions that allow function components to "hook into" React state and lifecycle features. Returning JSX from a custom hook elevates it to the level of a functional component, as it directly renders UI components.
10. What is the future of React hooks?
React hooks are still in the works, but they will be the future of React state management. They are more powerful and adaptable than class components and will most likely become the default approach to developing React components in the future.
Take our free react skill challenge to evaluate your skill
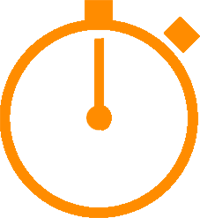
In less than 5 minutes, with our skill challenge, you can identify your knowledge gaps and strengths in a given skill.