27
JunUnit Testing in React
Introduction to Unit Testing in React
To assure the integrity and dependability of their code, developers must engage in unit testing. Unit testing in the context of React refers to testing distinct parts or sections of code. Devs can find and repair flaws early in the development process by isolating the components & testing them separately. The necessity of unit testing in React will be covered in detail, along with a step-by-step tutorial on how to create successful unit tests using well-liked testing frameworks like Jest et Enzyme. For comprehensive training on React and unit testing, consider enrolling in the Best React JS Course available for hands-on practice and deep knowledge.
The importance of unit testing
- In the software development lifecycle, unit testing is essential, especially for React apps.
- It assists developers in making sure that specific components are operating properly and producing the desired results.
- It will save time and effort in the future by detecting defects at the unit level and stopping them from spreading to other parts of the program.
- Unit tests provide information about the behavior of components and serve as living documentation.
- They simplify the codebase for developers to understand and manage.
Read More - React Interview Questions And Answers For Experienced
Understanding Unit Testing Tools and Jargon
We must become acquainted with the terminology and techniques used frequently in the industry before delving into the specifics of unit testing tools in React. Let's look at some essential unit testing tools ideas:
- Test Runner: A test runner is a program or framework that runs unit tests and produces reports. The React community makes extensive use of the well-liked test runner Jest.
- Test Suites: A test suite is a group of test cases or scenarios that pertain to one particular feature or component. Test suites are useful for successfully organizing and classifying tests.
- Assertions: Statements that support the predicted behavior of the tested code are known as assertions. They assist developers find inconsistencies by determining whether the output corresponds to the anticipated result.
- Mocks and Stubs: Mocks and stubs are used for replicating dependencies or external resources that a component depends on. They enable developers to test the component independently of how these dependencies are implemented.
What is jest in unit test
Jest is an effective JavaScript testing framework, https://jestjs.io/, jest testing React offers React developers a nice testing environment. jest testing react has a wide range of features, such as an easy-to-use API, an integrated test runner, and thorough documentation.
You must first create a new React project or access an existing project directory to begin using jest testing react
Install Jest using npm or yarn as a dev dependency:
npm install --save-dev jest
- Make a new directory called __tests__ at the project root level:
mkdir __tests__
- Create a file with the.test.js extension and name it MyComponent.test.js for your first unit test:
import { render, screen } from '@testing-library/react';
import MyComponent from '../MyComponent';
test('renders MyComponent without errors', () => {
render(<MyComponent />);
const componentElement = screen.getByTestId('my-component');
expect(componentElement).toBeInTheDocument();
});
The MyComponent is being rendered in the example above, and it is being claimed that there are no rendering issues. A render function from @testing-library/react enables us to recreate the rendering of a component, while screen.getByTestId helps us select elements based on their data-testid attribute.
Read More - React developer salary in India
Writing Unit Tests for React Components with Jest
Let's take a closer look at creating thorough unit tests for React components now that Jest has been configured and our first test has been created. To make sure that React components function as intended in diverse circumstances, it is essential to test them using a variety of scenarios and edge cases. Here are some recommendations for using Jest to create successful unit tests:
- Test Component Rendering: Check the component's rendering to make that it renders correctly and that the produced output contains all of the anticipated elements.
- Simulate User Interaction: Test user interactions by emulating actions including form submissions, clicks, and inputs. Check to see if the component reacts to these interactions correctly.
- Verify the component state: Examine how the state of a component changes by altering props or activating events. Check to see if the component appropriately re-renders and updates its state.
- Mock Dependencies: To simulate dependencies or external resources that the component depends on, use mocks and stubs. This enables you to test the component independently without depending on how these dependencies are implemented.
Introduction to enzyme test
Enzyme https://enzymejs.github.io/enzyme/ complements Jest by delivering extra utility methods and a more user-friendly API for testing React components, while Jest offers a strong testing framework for React applications. Component rendering, manipulation, and traversal are made simpler by Enzyme, which makes it simpler to build expressive and understandable tests.
Let's explore Enzyme's main attributes and how to incorporate them into your workflow for unit testing.
- Using npm or yarn, install Enzyme as a development dependency:
npm install --save-dev enzyme enzyme-adapter-react-16
- In your test setup file, such as setupTests.js, configure Enzyme as follows:
import { configure } from 'enzyme';
import Adapter from 'enzyme-adapter-react-16';
configure({ adapter: new Adapter() });
- Create a file with the.test.js extension and name it MyComponent.test.js to house your first Enzyme test:
import { shallow } from 'enzyme';
import MyComponent from '../MyComponent';
describe('MyComponent', () => {
test('renders without errors', () => {
const wrapper = shallow(<MyComponent />);
expect(wrapper.exists()).toBe(true);
});
});
The shallow function from Enzyme is used in the example above to render MyComponent without rendering any of its child components. The component is then declared to be present in the displayed output.
Writing Unit Tests for React Components with Enzyme
Enzyme test provides a rich set of utility functions that make it easy to write comprehensive unit tests for React components. Here are some common scenarios and techniques you can use when writing unit tests with Enzyme:
- Finding and Selecting Elements: Use Enzyme's find, filter, and at methods to select elements within the rendered component. You can use CSS selectors, component names, or other attributes to locate elements.
- Simulating Events: Use Enzyme's simulate method to trigger events on elements. You can simulate various events like clicks, inputs, and form submissions to test the component's behavior.
- Checking Prop Values: Use Enzyme's props method to access and assert the values of props passed to the component. Verify that the component receives the expected props and handles them correctly.
- Testing Component Lifecycle Methods: Use Enzyme's mount function to fully render the component and test its lifecycle methods such as componentDidMount and componentDidUpdate.
Common Challenges and How to Overcome Them in Unit Testing React
Let's explore some typical difficulties and talk about solutions:
- Testing Externally Dependent Components: Writing isolated unit tests for a component that depends on external dependencies like APIs or libraries might be difficult. When this occurs, think about simulating these dependents' behavior via mocks or stubs.
- Component Async Operation Testing: It can be challenging to test components that use timers or asynchronous API calls. To handle async actions in your tests, use react-testing-library or the built-in async testing support in Jest.
- Maintaining Test Coverage: As your software expands, maintaining adequate test coverage can be difficult. Review your test suite frequently and note any areas that need more testing. To keep track of your tests' coverage, think about employing code coverage tools.
- Testing UI Interactions: Testing user interfaces with drag-and-drop functionality or intricate workflows can be challenging. Consider creating end-to-end tests that mimic user interactions using software such as Cypress or the React Testing Library.
Best Practices for Unit Testing in React
Let's talk about a few principles that should be followed when creating unit tests for React applications:
- Keeping Tests Separate: Make sure each unit test is independent and does not depend on the outcome or side effects of other tests. Debugging is made easier and test reliability is maintained as a result.
- Discuss edge cases: To make sure your components can handle a range of inputs and situations, test multiple scenarios and edge cases. Examining boundary values, empty states, & mistake situations are some examples.
- Utilise descriptive test names: To promote readability and maintainability, give your test cases titles that are descriptive and simple. A test case with a clear name serves as documentation that helps in the comprehension of the test's objective by other developers.
- Update Tests Frequently: As your codebase develops, be careful to update your unit tests. To make sure they appropriately reflect the behavior of the code, rework your tests at the same time refactor your components.
Resources for further learning and practice
- Official Redux documentation: Read the documentation at https://legacy.reactjs.org/docs/getting-started.html The official Redux documentation is a comprehensive resource that covers all aspects of Redux, including React Redux.
- Online tutorials and courses: Explore Additional Learning and Practice Resources with us at https://www.scholarhat.com/training/reactjs-certification-training. There are numerous online tutorials and courses available that specifically focus on React Redux.
Summary
With the help of well-known testing frameworks like Jest including Enzyme, we have presented a step-by-step tutorial and an in-depth analysis of the importance of unit testing in React. We have discussed key ideas, best practices, and typical difficulties while unit testing React components. You may enhance the quality and dependability of your React applications, find defects early in the development process, and guarantee a positive user experience by becoming an expert at unit testing. To fully realize the potential of your React applications, start integrating unit testing into your workflow right away.
Take our React skill challenge to evaluate yourself!
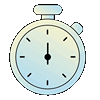
In less than 5 minutes, with our skill challenge, you can identify your knowledge gaps and strengths in a given skill.