Redux in React
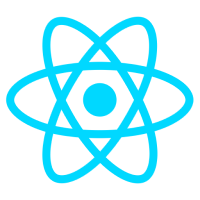
ReactJS Course
Introduction
Due to its capacity to streamline state management and improve the functionality of web applications, React Redux has grown in popularity among web developers. In this article, we'll look at React Redux's many facets and discover how to use it to improve web development. This step-by-step manual will help you realize the full potential of React Redux, whether you are a beginner or have some prior experience. For a comprehensive understanding and hands-on experience with React Redux, consider enrolling in React JS Certification Training to master this powerful combination of technologies.
What is redux in react
For JavaScript applications, Redux is a predictable state management library that is frequently used with React. It offers a central location to keep track of an application's state and facilitates quick state updates via actions and reducers, encouraging a one-way data flow.
Why do we use redux in React?
- JavaScript applications can use the Redux state management library.
- It offers a predictable state container, which makes managing the state between components simpler.
- Redux streamlines data flow and ensures consistent handling of changes by centralizing the state in a single store.
- Redux's predictability promotes faster efficiency, better debugging, and more maintainable code.
- Redux is frequently used in React applications to manage complex states and works nicely with React.
Read More - React Interview Questions For Freshers
Benefits of Using React Redux in Web Development
React Redux is a popular option for web developers due to the many advantages it provides:
- State management that is predictable: React Redux offers a predictable state management framework, making it simpler to comprehend and debug code. It ensures consistent and effective management of state changes by centralizing the application state in a single store
- Reusable parts: React Redux encourages the idea of reusable parts, which are easily transferable between other application components. As a result, development time and effort are decreased while code organization and reusability are improved.
- Performance improvement: To improve performance, React Redux makes use of a virtual DOM. When the state changes, it effectively updates only those components that are required, reducing the amount of DOM manipulations. The user experience is enhanced and rendering is accelerated as a result.
React Redux tutorial: Setting up a basic project
Let's first put up a simple project before we get into the fundamental ideas of React Redux. You must have Node.js & npm installed on your computer to follow along with this tutorial.
- Create a new directory for your project to begin with, then use the command line to enter it.
- To start a new npm project, enter the following command:
npm init
cRun the following command to install the required dependencies:
npm install react react-dom redux react-redux
- In the project's root directory, make a new file called index.js, and then add the following code:
import React from 'react';
import ReactDOM from 'react-dom';
import { Provider } from 'react-redux';
import { createStore } from 'redux';
import App from './App';
import rootReducer from './reducers';
const store = createStore(rootReducer);
ReactDOM.render(
<Provider store={store}>
<App />
</Provider>,
document.getElementById('root')
);
- In the project's root directory, create a new file called App.js, and then add the following code:
import React from 'react';
import { connect } from 'react-redux';
const App = (props) => {
return (
<div>
<h1>Welcome to React Redux Tutorial</h1>
<p>Current count: {props.count}</p>
<button onClick={props.increment}>Increment</button>
<button onClick={props.decrement}>Decrement</button>
</div>
);
};
const mapStateToProps = (state) => {
return {
count: state.count,
};
};
const mapDispatchToProps = (dispatch) => {
return {
increment: () => dispatch({ type: 'INCREMENT' }),
decrement: () => dispatch({ type: 'DECREMENT' }),
};
};
export default connect(mapStateToProps, mapDispatchToProps)(App);
- In the project's root directory, create a new file called reducers.js, and then add the following code:
const initialState = {
count: 0,
};
const rootReducer = (state = initialState, action) => {
switch (action.type) {
case 'INCREMENT':
return { count: state.count + 1 };
case 'DECREMENT':
return { count: state.count - 1 };
default:
return state;
}
};
export default rootReducer;
- Finally, add the following code to an HTML file called index.html in the root directory:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>React Redux Tutorial</title>
</head>
<body>
<div id="root"></div>
<script src="index.js"></script>
</body>
</html>
Read More - React developer salary
Understanding the core concepts of React Redux
React Redux's fundamental ideas must be understood to be used effectively.
- Store: At its core, React Redux revolves around the concept of a store, which houses the application state. A Redux library method called createStore is used to create the store.
- Immutable State: In React Redux, the state should be considered read-only. It shouldn't be changed directly. State changes are instead implemented via actions, which are basic JavaScript objects that specify the kind of change to be done.
- Reducers: Reducers are used to change the state. Reducers are pure functions that take the input of the current state and an action and output a new state. It is significant to highlight that reducers should never modify the existing state object; instead, they should always return a new one.
- Provider: React Redux offers a Provider component that makes the application's store available to all components. We guarantee that all child components may access the store by wrapping the root component in the Provider component and passing the store as a prop.
Container component vs presentational component
Container component vs presentational components is introduced in React Redux to effectively isolate UI rendering from state management:
- Presentational Components: They are in charge of rendering the user interface and capturing user input. They frequently obtain the necessary data and callbacks through props and are stateless. Presentational components are primarily concerned with appearance and are unaware of Redux or the application state.
- Container Components: These components are linked to the Redux store and are in charge of controlling the state and behavior of the application. They offer information as well as callbacks to presentational elements. The functional focus of container components interacts with the Redux store.
- Reusability and maintainability are enhanced by the separation of presentational and container components. While container components offer a centralized area for maintaining the state, presentational components can be utilized throughout the application.
Utilizing high order components in react
- High order components in React (HOCs) are a strong component composition and code reuse feature of React Redux. HOCs are procedures that accept an input component and output a new component with more features.
- High order components in react redux are frequently used to give components that are not directly related to the store access to the Redux store and state information. This may be accomplished by using React Redux's connect method, a HOC.
- MapStateToProps and mapDispatchToProps are two optional arguments that the connect function accepts. The function mapStateToProps allows a component to access the required data by mapping the component's props to the component's state from the Redux store. The function mapDispatchToProps allows a component to dispatch actions to the Redux store by mapping the dispatch function to the component's props. We can quickly improve the functionality of our components while making them more adaptable and reusable by using HOCs and the connect function.
React redux map state to props and map Dispatch To Props: How to use them effectively
- The utilities react redux map state to props and map Dispatch To Props are essential for tying our components to the Redux store to manage the state.
- The Redux store's state is used as the input for the mapStateToProps method, which outputs an object that maps the state to the component's props. As a result, the component can have access to the required data from the store.
const mapStateToProps = (state) => {
return {
count: state.count,
};
};
- The mapStateToProps function in the example above converts the state's count value to the component's count prop. On the other side, the map Dispatch To Props method allows a component to dispatch actions to the Redux store by mapping the dispatch function to the component's props.
const mapDispatchToProps = (dispatch) => {
return {
increment: () => dispatch({ type: 'INCREMENT' }),
decrement: () => dispatch({ type: 'DECREMENT' }),
};
};
- The react redux map state to props function in the example above allows the component to dispatch the INCREMENT & DECREMENT actions by mapping the increment & decrement functions to the appropriate component props.
Real-world examples of React Redux in action
Let's look at some real-world instances of React Redux being used effectively so that you can better grasp how it may be used in practice.
- E-commerce sites: To handle user identification, manage the shopping cart, and monitor orders, many e-commerce websites employ React Redux. React Redux's centralized state management makes complicated operations simple and guarantees a seamless user experience.
- Social media applications: Real-time updates and intricate user interactions are frequently needed for social media applications. The quick presentation of posts, comments, and notifications is made possible using React Redux, which is perfectly suited for handling the state of social media applications.
- Dashboard and analytics tools: Real-time data updates and dynamic visualizations are necessary for dashboard and analytics tools. React Redux is the best option for creating such applications since it offers a solid foundation for managing state and rendering dynamic data.
These are just a few situations where React Redux can be used in the real world. React Redux is a strong solution for a variety of web development projects because of its flexibility and scalability. To harness the full potential of React Redux and become proficient in its implementation, consider enrolling in the Best React JS Course available for in-depth training and practical knowledge.
Best practices for using React Redux
Follow these guidelines for clear and maintainable code while using React Redux:
- Normalize state: Maintain a normalized state in the Redux store to enhance efficiency and make state management simpler. Don't nest objects or arrays deeply.
- Use selectors: Use selectors to encapsulate the logic for gaining access to particular areas of the state. Separating components from the state structure, improves the readability and maintainability of the code.
- Stay away from needless re-renders: Reduce re-renders to improve performance. Implement shouldComponentUpdate in container components or use memoization strategies.
- Separate business logic from presentation: By separating business logic from presentation elements, you can improve code organization. This improves reusability and testability.
- Observe the name conventions: Keep the names of your actions, reducers, and selectors consistent. This enhances the readability of the code and makes it easier for engineers to collaborate.
Resources for further learning and practice
- Official Redux documentation: Read the documentation at https://legacy.reactjs.org/docs/getting-started.html The official Redux documentation is a comprehensive resource that covers all aspects of Redux, including React Redux.
- Online tutorials and courses: Explore Additional Learning and Practice Resources with us at https://www.scholarhat.com/training/reactjs-certification-training. There are numerous online tutorials and courses available that specifically focus on React Redux.
Summary
A strong technology called React Redux makes state management easier and improves the functionality of web apps. You have learned how to build up a fundamental React Redux project, comprehend the fundamental ideas, work with higher-order components, and efficiently use mapStateToProps and mapDispatchToProps by following this step-by-step tutorial. Insights into best practices, real-world examples, and resources for more learning and practice have also been garnered.
Take our free react skill challenge to evaluate your skill
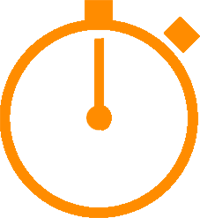
In less than 5 minutes, with our skill challenge, you can identify your knowledge gaps and strengths in a given skill.