Conditional Rendering in React: Creating Dynamic User Interfaces
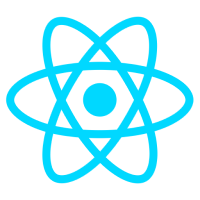
ReactJS Course
Introduction
Developers may design dynamic and interesting user interfaces using React's advanced conditional rendering method. You may control when and what material is rendered using this strategy, which enhances user experience and results in simpler code and more adaptable components. To master the art of conditional rendering and other advanced React techniques, consider enrolling in the Best React course available.
What is Conditional Rendering?
React's core concept of conditional rendering enables you to display or hide items depending on specific circumstances. It is frequently employed to manage user interactions, such as presenting a popup when a button is pressed or displaying various content depending on the user's authentication state. You may dynamically adjust the user interface and offer a more individualized experience by using conditional rendering.
The following are the basics of React's conditional rendering:
- The conditional operator and other JavaScript operators are used to render conditions.
- Depending on the state of your application, you can use conditional rendering to render various components, elements, or texts.
- It is possible to implement access levels, toggle program functionality, and show or conceal items using conditional rendering.
- It's necessary to utilize conditional rendering carefully because it can complicate your code.
- Make sure your code is still productive even when conditional rendering is used.
Read More - Top 50 Mostly Asked React Interview Question & Answers
Implementing Conditional Rendering
There are numerous ways to achieve conditional rendering in React. Each technique has its own syntax and usage cases. Let's study the most popular techniques:
- The if/else Statement: React's if/else statement functions similarly to JavaScript's treatment of circumstances. React will alter the user interface in accordance with elements created using JavaScript operators like if and else.
Here's an example:
import React from 'react';
const ExampleComponent = ({ isLoggedIn }) => {
if (isLoggedIn) {
return <h1>Welcome, User!</h1>;
} else {
return <h1>Please log in to continue.</h1>;
}
};
export default ExampleComponent;
The isLoggedIn prop is sent to the ExampleComponent component so it may determine whether the user is logged in. According to the isLoggedIn value, it employs an if/else statement to render the headings "Welcome, User!" if true and "Please log in to continue" if false. In React, conditional rendering is a standard technique.
- The Ternary Operator: Another often used technique for conditional rendering in React is the ternary operator. It enables simpler conditionally rendering of items.
Here's an example:
import React from 'react';
const ExampleComponent = ({ isLoggedIn }) => (
<div>
{isLoggedIn ? (
<h1>Welcome, User!</h1>
) : (
<h1>Please log in to continue.</h1>
)}
</div>
);
export default ExampleComponent;
The isLoggedIn prop is sent to the ExampleComponent component so it may determine whether the user is logged in. It renders several JSX expressions depending on the value of isLoggedIn using the ternary operator isLoggedIn? (...): (...): "Welcome, User!" if true and "Please log in to continue" if false. This is a typical method for conditional rendering in React.
- Inline IF with Logical && Operator: React supports inline conditional rendering using the logical && operator. Based on the criteria, you can use it to conditionally include or exclude a JSX element. Here's an example:
import React from 'react';
const ExampleComponent = ({ isLoggedIn }) => (
<div>
{isLoggedIn && <h1>Welcome, User!</h1>}
</div>
);
export default ExampleComponent;
The isLoggedIn prop is sent to the ExampleComponent component so it may determine whether the user is logged in. It executes the inline if statement along with the logical && operator to conditionally render the JSX expression <h1>Welcome, User!</h1> if isLoggedIn is true. React components can render under certain conditions thanks to this short method.
- Switch Case Operator: React's switch case operator can be used for conditional rendering scenarios that are more complicated. It enables you to manage various situations and render various components based on particular circumstances.
Here's an example:
import React from 'react';
const MyComponent = ({ status }) => {
let componentToRender;
switch (status) {
case 'loading':
componentToRender = <div>Loading...</div>;
break;
case 'success':
componentToRender = <div>Success!</div>;
break;
case 'error':
componentToRender = <div>Error occurred.</div>;
break;
default:
componentToRender = <div>Unknown status.</div>;
}
return (
<div>
<h1>Conditional Rendering Example</h1>
{componentToRender}
</div>
);
};
export default MyComponent;
A status prop is used by the MyComponent to render various components. It uses a switch case to verify the prop value before assigning the JSX component and rendering it. This makes status-based conditional rendering possible.
- Conditional Rendering with Enums: Enums give JavaScript programmers a means to define a collection of named constants. Enums can be used in React conditional rendering to provide more organized and legible code. Here's an example:
import React from 'react';
const StatusEnum = {
LOADING: 'loading',
SUCCESS: 'success',
ERROR: 'error'
};
const MyComponent = ({ status }) => {
let componentToRender;
switch (status) {
case StatusEnum.LOADING:
componentToRender = <div>Loading...</div>;
break;
case StatusEnum.SUCCESS:
componentToRender = <div>Success!</div>;
break;
case StatusEnum.ERROR:
componentToRender = <div>Error occurred.</div>;
break;
default:
componentToRender = <div>Unknown status.</div>;
}
return (
<div>
<h1>Conditional Rendering Example</h1>
{componentToRender}
</div>
);
};
export default MyComponent;
To represent status values, a StatusEnum object is created. The component to render is chosen by MyComponent using the status attribute. The switch case enables conditional rendering after comparing the prop to the enum values and assigning the JSX component appropriately.
- Higher-Order Components: React's Higher-Order Components (HOC) pattern lets you reuse component logic. By enclosing components in additional logic, they can also be utilized for conditional rendering. HOCs offer a technique to improve components with certain circumstances and behaviors.
Here's an example:
import React from 'react';
const withConditionalRendering = (WrappedComponent) => {
return ({ condition, ...props }) => {
if (condition) {
return <WrappedComponent {...props} />;
} else {
return null;
}
};
};
const MyComponent = ({ name }) => {
return <div>Hello, {name}!</div>;
};
const ConditionalComponent = withConditionalRendering(MyComponent);
const App = () => {
const showComponent = true;
return (
<div>
<h1>Conditional Rendering Example</h1>
<ConditionalComponent condition={showComponent} name="John" />
</div>
);
};
export default App;
WithConditionalRendering is a HOC that we define that accepts a wrapped component and renders it conditionally based on a condition prop. Using withConditionalRendering, we create ConditionalComponent, which displays a greeting on MyComponent. We render ConditionalComponent with a name prop conditionally in App. If you're aiming to solidify your React skills and gain recognition for your expertise, consider pursuing a React JS Certification to showcase your proficiency in React development.
Read More - React developer salary in India
Best Practices for Conditional Rendering
To ensure clean and maintainable code while implementing conditional rendering in React, best practices must be followed. Here are some concepts to think about:
- Select the best technique for each use case: Different conditional rendering techniques might be necessary for various contexts. Consider your application's requirements before selecting the approach that will work best.
- Maintainable and tidy code: If not properly organized, conditional rendering might grow complicated. To make your code simpler to understand and maintain, use clear and concise naming conventions, divide functionality into reusable functions or components, and document your code.
- Test as well as debug conditional rendering logic: As using any code, it's essential to test as well as debug the conditional rendering logic completely. Make sure all conceivable outcomes are taken into account, and deal with any edge situations or potential mistakes.
Resources for further learning and practice
- Official Redux documentation: Read the documentation at https://legacy.reactjs.org/docs/getting-started.html The official Redux documentation is a comprehensive resource that covers all aspects of Redux, including React Redux.
- Online tutorials and courses: Explore Additional Learning and Practice Resources with us at https://www.scholarhat.com/training/reactjs-certification-training . There are numerous online tutorials and courses available that specifically focus on React Redux.
Conclusion
React's conditional rendering approach is a potent tool for building dynamic and interesting user interfaces. You can give your consumers a more customized and interactive experience by rendering components only when certain criteria are met. The if/else statement, ternary operator, inline IF with logical && operator, switch case operator, conditional rendering using enums, and higher-order components are just a few of the conditional rendering techniques we looked at in this post for React. Additionally, we went through how to utilize the useState hook to handle the application state for conditional rendering, and we showed actual examples of conditional rendering utilizing props. You may improve the flexibility and adaptability of your React applications by adhering to recommended practices and using these strategies successfully.
Take our free react skill challenge to evaluate your skill
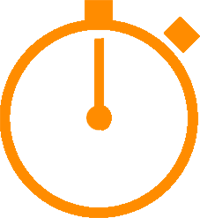
In less than 5 minutes, with our skill challenge, you can identify your knowledge gaps and strengths in a given skill.