Objects and Classes in C#: Examples and Differences
Objects and Classes in C#: An Overview
C# (pronounced C sharp) is a versatile and powerful programming language that was developed by Microsoft. It is widely used in various applications, including web development, game development, and desktop applications. In this article, we will explore the concept of objects and classes in C#, and understand how they play a crucial role in the programming language.
What is a Class in C#?
In C#, a class is a blueprint for creating objects and defining their properties, methods, and events. It acts as a template, encapsulating data and behavior. Classes enable object-oriented programming, promoting code reusability and organization by grouping related functionality. Instances of classes are used to create objects in C# programs.
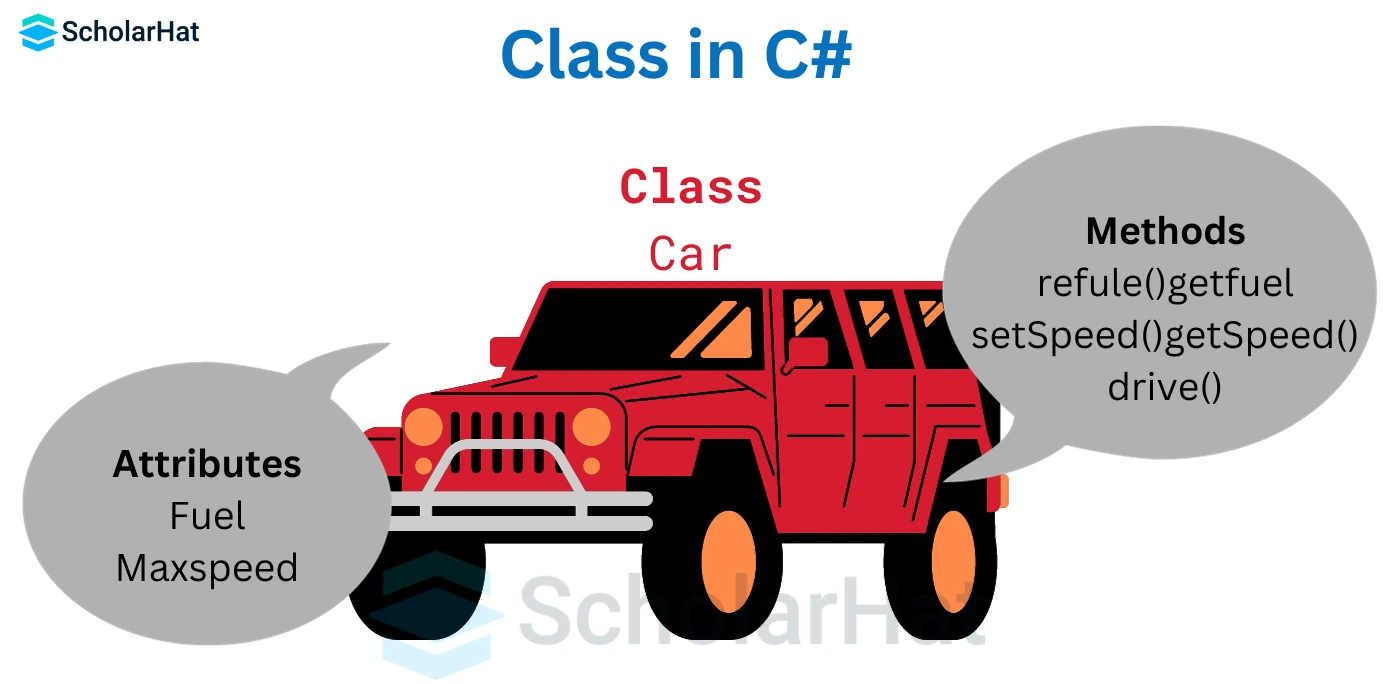
Declaration of Class
A class declaration typically starts with the term class and is followed by the identifier (name) of the class. But depending on the needs of the application, some optional attributes can be used with class declaration.
- Modifiers: A class may be internal, public, etc. The class's default modifier is internal.
- Keyword class: The type class is declared using the class keyword.
- Class Identifier: There is a class variable available. The first letter of the identifier, also known as the class name, should always be capitalized.
- Base class or Super class: the name of the class's parent (superclass), if one exists, followed by a colon. This is an optional step.
- Interfaces: a list of the interfaces that the class has implemented, separated by commas and beginning with a colon (if any). Multiple interfaces may be implemented by a class. You can omit this.
- Body: There are curly braces all around the class body.
New objects are initialized in class constructors. While methods are used to implement the behavior of the class and its objects, fields are variables that give the state of the class and its objects.
// declaring public classpublic class Scholar
{
// field variable
public int a, b;
// member function or method
public void display()
{
Console.WriteLine(“Class & Objects in C#”);
}
}
Read More - C# Interview Questions And Answers
What is an Object in C#?
In C#, an object is a fundamental data type that represents a real-world entity. It combines data (variables) and methods (functions) that operate on the data, encapsulating behavior and state. Objects are instances of classes, defining their structure and behavior, facilitating the principles of object-oriented programming in C#.
An object consists of:
- State: It is represented by attributes of an object. It also reflects the properties of an object.
- Behavior: It is represented by the methods of an object. It also reflects the response of an object with other objects.
- Identity: It gives a unique name to an object and enables one object to interact with other objects.
Consider Person as an object and see the below diagram for its identity, state, and behavior.
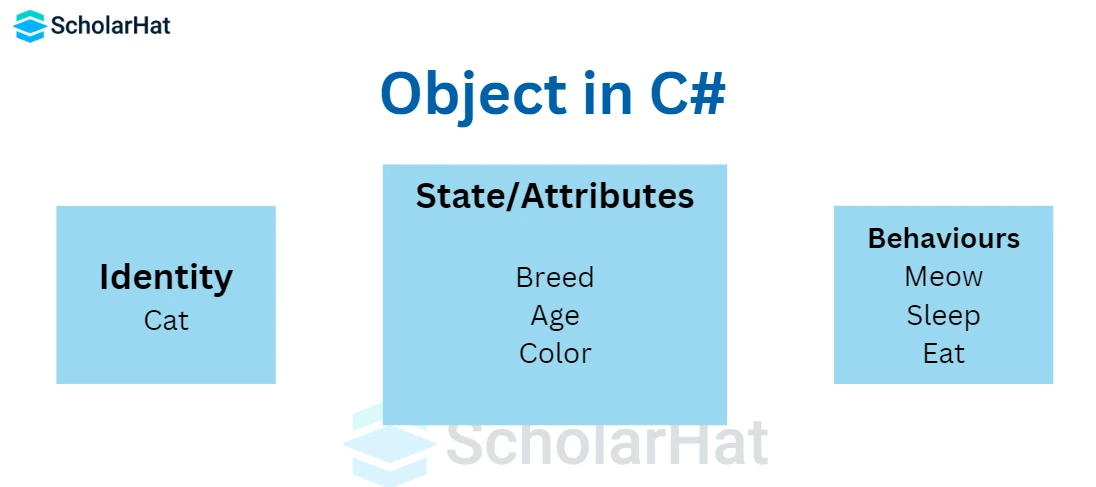
Declaring Objects
A class is said to be instantiated when an instance of the class is created. The characteristics and actions of the class are shared by all instances. However, each object has a different value for these characteristics, which is the state. Any number of instances can be found within a class.
Objects refers to things present in the real world. For example, a shapes program may have objects such as “triangle”, “square”, “circle”. An online learning system might have objects such as “student”, and “course”.
As we declare variables, we use the syntax (type name;). This tells the compiler that the name will be used to refer to data with the type type. This declaration additionally sets aside the necessary amount of memory for the variable when it is a primitive one. Consequently, a reference variable's type must strictly be a concrete class name.
Person Employee;
When a reference variable (Employee) is declared in this way, its value will be null until an actual object is created and assigned to it. An object cannot be created by merely declaring a reference variable.
Initializing an Object
A class is created by the new operator, which allocates memory for a new object and then returns a reference to that memory. Additionally, the new operator calls the class constructor.
Example
using System;
class Person
{
public string Name { get; set; }
public int Age { get; set; }
}
class Program
{
static void Main()
{
// Initializing an object of the Person class
Person person = new Person();
// Setting properties of the object
person.Name = "Employee";
person.Age = 25;
// Displaying object properties
Console.WriteLine("Name: " + person.Name);
Console.WriteLine("Age: " + person.Age);
}
}
Explanation
In this example in the C# Compiler, we create a Person class with Name and Age properties. In the Main method, we create an instance of the Person class, set its properties, and then display the values. This demonstrates the basic process of initializing and working with objects in C#.
Output
Name: Employee
Age: 25
Difference between Objects and Classes in C#
Objects | Classes |
An object is an instance of a class. | A class is a blueprint or a template that defines the structure and behavior of objects |
It represents a specific entity or an individual occurrence based on the class definition. | It encapsulates data (attributes/properties) and functions (methods) that operate on that data. |
Objects have state (values of attributes/properties) and behavior (methods). | Classes define the common characteristics shared by multiple objects. |
They are created using the new keyword, which allocates memory and initializes the object. | They serve as a blueprint for creating objects. |
Objects can interact with each other by invoking methods or accessing properties. | Classes are defined using the class keyword. |
Example of Objects and Classes in C#
Example:1
using System;
class BankAccount
{
private string accountNumber;
private decimal balance;
public BankAccount(string accountNumber, decimal balance)
{
this.accountNumber = accountNumber;
this.balance = balance;
}
public void Deposit(decimal amount)
{
balance += amount;
}
public void Withdraw(decimal amount)
{
if (amount <= balance)
{
balance -= amount;
}
else
{
Console.WriteLine("Insufficient funds.");
}
}
public void PrintBalance()
{
Console.WriteLine($"Account Number: {accountNumber}");
Console.WriteLine($"Balance: {balance:C}");
}
}
class Program
{
static void Main(string[] args)
{
BankAccount myAccount = new BankAccount("123456789", 1000);
myAccount.PrintBalance();
myAccount.Deposit(500);
myAccount.PrintBalance();
myAccount.Withdraw(200);
myAccount.PrintBalance();
myAccount.Withdraw(2000); // This will result in "Insufficient funds" message
myAccount.PrintBalance();
}
}
Explanation
This C# program in the C# Compiler defines a BankAccount class with private fields for account number and balance. It has methods for depositing, withdrawing, and printing the account balance. In the Main method, it creates an instance of BankAccount, performs deposit and withdrawal operations, and prints the balance. If a withdrawal exceeds the balance, it displays an "Insufficient funds" message.
Output
Account Number: 123456789
Balance: $1,000.00
Account Number: 123456789
Balance: $1,500.00
Account Number: 123456789
Balance: $1,300.00
Insufficient funds.
Account Number: 123456789
Balance: $1,300.00
Example: 2
using System;
// Define a class called "Person"
class Person
{
// Class fields/properties
public string Name { get; set; }
public int Age { get; set; }
// Class constructor
public Person(string name, int age)
{
Name = name;
Age = age;
}
// Class method
public void SayHello()
{
Console.WriteLine($"Hello, my name is {Name} and I am {Age} years old.");
}
}
class Program
{
static void Main(string[] args)
{
// Create a new instance of the Person class
Person person1 = new Person("John", 25);
// Access the properties of the person1 object
Console.WriteLine($"Person 1: Name - {person1.Name}, Age - {person1.Age}");
// Call the SayHello method of person1
person1.SayHello();
// Create another instance of the Person class
Person person2 = new Person("Jane", 30);
// Access the properties of the person2 object
Console.WriteLine($"Person 2: Name - {person2.Name}, Age - {person2.Age}");
// Call the SayHello method of person2
person2.SayHello();
}
}
Explanation
This C# program defines a Person class with properties for name and age, a constructor to initialize these properties, and a SayHello method to print a greeting message. In the Main method, it creates two Person objects, sets their properties, and calls the SayHello method to display their information.
Output
Person 1: Name - John, Age - 25
Hello, my name is John and I am 25 years old.
Person 2: Name - Jane, Age - 30
Hello, my name is Jane and I am 30 years old.
Conclusion
Object in C# and class in c# are fundamental concepts in C# programming that enable developers to create efficient and modular code. By understanding and harnessing the power of objects and classes, developers can unlock the full potential of C# and create software solutions that meet the ever-evolving needs of the industry.
Take our Csharp skill challenge to evaluate yourself!
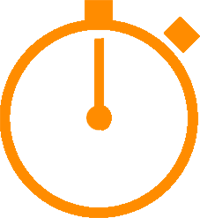
In less than 5 minutes, with our skill challenge, you can identify your knowledge gaps and strengths in a given skill.