26
OctExtension Methods in C#: Types, Real-Time Examples
C# Extension method
What Are Extension Methods?
- An extension method is a special kind of static method that allows you to add new functionality to existing types without modifying them.
- You can use them on classes, structs, or even interfaces.
- When you write an extension method, it behaves like an instance method of the type being extended, making your code more readable and organized.
- Extension methods are defined as static methods in a static class, and the first parameter specifies the type being extended, prefixed by this keyword.
When to Use an Extension Method
There are certain scenarios where extension methods are especially useful.
- Third-party Libraries: If you're working with a class from a third-party library that you cannot modify, an extension method allows you to add extra functionality.
- Adding Utility Functions: If a method doesn't logically belong to a specific class, you can use an extension method to add it to a utility class.
- Simplifying Code: They can help reduce boilerplate code by adding methods that make common tasks simpler.
Syntax of an Extension Method
- An extension method must be defined in a static class.
- The method itself must be static.
- The first parameter of the method specifies which type the extension method is extending.
- This parameter must be preceded by the "this" keyword.
How Extension Methods Work
- Extension methods must be declared inside a static class.
- The method itself must be static.
- The first parameter in the method defines which type it extends and must be preceded by the "this" keyword.
Let’s understand this with a simple example.
Example
using System;
public static class StringExtensions
{
// Extension method to count words in a string
public static int WordCount(this string str)
{
if (string.IsNullOrWhiteSpace(str))
{
return 0;
}
return str.Split(' ').Length;
}
}
public class Program
{
public static void Main()
{
string sentence = "Hello, this is a simple sentence.";
int count = sentence.WordCount(); // Using the extension method
Console.WriteLine("Word Count: " + count); // Output: Word Count: 6
}
}
Output
Output:
Word Count: 6
Explanation
- The method WordCount is static and belongs to the StringExtensions class.
- The this string str parameter specifies that the method extends the string type.
- You can now call WordCount() on any string as if it were a method of the string class itself.
Create a Class Library
Create a Class Library project in Visual Studio. This class will represent a library containing a basic class with some methods. Here’s the code for the class:
Code for Class Library (MathLib):
using System;
namespace MathLib
{
public class Calculator
{
public int Add(int a, int b)
{
return a + b;
}
public int Multiply(int a, int b)
{
return a * b;
}
}
}
Create a Console Application
Next, create a Console Application project in Visual Studio. This application will reference the MathLib class library and will contain an extension method to extend the functionality of the Calculator class.
- Add a reference to the MathLib class library in your console application.
- Then, use the following code for the console application.
Example: Code for Console Application (ExtensionMethodDemo)
using System;
using MathLib; // Reference the class library
namespace ExtensionMethodDemo
{
// Static class for extending Calculator
public static class CalculatorExtensions
{
// Extension method to calculate the square of a number
public static int Square(this Calculator calculator, int number)
{
return number * number;
}
}
class Program
{
static void Main(string[] args)
{
// Create an instance of Calculator
Calculator calc = new Calculator();
// Use the existing methods from MathLib
Console.WriteLine("Addition: " + calc.Add(10, 5));
Console.WriteLine("Multiplication: " + calc.Multiply(10, 5));
// Use the new extension method Square
int number = 6;
Console.WriteLine("Square of " + number + ": " + calc.Square(number));
Console.ReadKey();
}
}
}
Explanation
- The Calculator class has two basic methods: Add and Multiply. It is a simple calculator for performing arithmetic operations.
- The CalculatorExtensions static class adds an extension method named Square to the Calculator class.
- This extension method takes the Calculator object as its first parameter (this Calculator calculator) and allows calculating the square of a number.
- In the Program class, the Calculator object (calc) calls its built-in methods (Add, Multiply) as well as the new extension method (Square).
Output
Addition: 15
Multiplication: 50
Square of 6: 36
Creating an Extension Method
Now, let's talk about how you would create an extension method.
- Define a Static Class: This class will hold your extension methods.
- Add a Static Method: The method should start with the "this" keyword in the parameter list to extend an existing type.
Example
In this example, we'll extend the string class to add a ReverseString method.
using System;
public static class StringExtensions
{
// Extension method to reverse a string
public static string ReverseString(this string str)
{
char[] charArray = str.ToCharArray();
Array.Reverse(charArray);
return new string(charArray);
}
}
public class Program
{
public static void Main()
{
string original = "Hello";
string reversed = original.ReverseString(); // Using the extension method
Console.WriteLine("Original: " + original); // Output: Original: Hello
Console.WriteLine("Reversed: " + reversed); // Output: Reversed: olleH
}
}
Output
Output:
Original: Hello
Reversed: olleH
Explanation
- The method ReverseString takes in a string and reverses the characters.
- The "this" keyword allows you to call ReverseString directly on any string instance, making it look like a built-in method.
Extension Methods Chaining
Like instance methods, extension methods also have the capability of chaining.
public static class ExtensionClass
{
public static string Pluralize (this string s) {...}
public static string Capitalize (this string s) {...}
}
// we can do chaining of the above methods like as
string x = "Products".Pluralize().Capitalize();
Ambiguity, resolution, and precedence of extension method
An extension method should be used in the same scope as the class. If two extension methods have the same signature, the more specific method takes precedence.
static class StringExtension
{
// first method
public static bool IsCapitalized(this string s) {...}
}
static class ObjectExtension
{
// second method
public static bool IsCapitalized(this object s) {...}
}
// code here
// first method is called
bool flag1 = "Dotnet-Tricks".IsCapitalized();
// second method is called
bool test2 = (ObjectHelper.IsCapitalized("Dotnet-Tricks"));
3. Seamless Integration
- You can use extension methods with both built-in and custom types, making them highly versatile.
- They integrate smoothly with your existing code and follow the same syntax as instance methods.
4. Reusability
- Once written, extension methods can be reused across multiple projects.
- They promote code reusability by encapsulating functionality in a single, easily maintainable location.
Limitations of Extension Methods
- Cannot Override Existing Methods: Extension methods can’t override existing methods in a class. If a type already has a method with the same name, the instance method will take precedence.
- Can Lead to Misuse: Overusing extension methods can clutter your codebase with methods that don’t belong to certain types. Use them wisely and avoid creating too many unrelated methods.
- Performance: While the performance hit of using extension methods is minimal, over-reliance on them for basic tasks can sometimes lead to inefficiencies.
Common Scenarios for Extension Methods
- String Manipulation: You can add methods for trimming, formatting, or parsing strings.
- LINQ-style Queries: LINQ itself relies on extension methods for query syntax. You can extend this further by adding your own query methods.
- Validation Methods: Extension methods are great for adding validation methods to types, such as checking if a string is an email or a URL.
- Collections: Adding methods to collections like List<T> to perform operations like checking for null or empty lists.
Summary
C# Extension Methods enable developers to improve the functionality of existing types without changing the original source code, making the code more legible and maintainable. Defined as static methods in a static class, they allow you to elegantly add new functionality to built-in types or third-party libraries. While they offer major benefits such as increased code flexibility, readability, and reusability, they must be used sparingly to prevent cluttering your codebase. To master theC# concept, enroll now in Scholarhat's C# Programming Course.
FAQs
Q1. What is extension method in C#?
Q2. What are extends C# methods?
Q3. What is the extension method contains in C#?
Take our Csharp skill challenge to evaluate yourself!
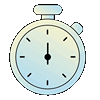
In less than 5 minutes, with our skill challenge, you can identify your knowledge gaps and strengths in a given skill.