14
JunUnderstanding Delegates in C#
Delegates in C#
Delegates in C# are types that define references to methods with a particular parameter list and return type. A delegate allows you to pass methods as arguments to other methods, making your code more flexible and reusable. They are commonly used for event handling and callback methods in C#. If you’re looking to master C# delegates, it’s crucial to understand their role in event-driven programming, their syntax, and how they allow methods to be assigned dynamically during runtime.
In this Csharp tutorial, we will dive deep into delegates in C# and explore their practical usage in different scenarios to enhance your programming skills.
To deepen your understanding and enhance your skills, you can enroll in a C Sharp Course Free and start learning today!
Read More: C# Interview Questions and Answers |
What is a Delegate in C#?
A delegate in C# is a type-safe function pointer that allows you to pass methods as parameters. It defines the signature of a method and holds a reference to a method with a matching signature, making it possible to invoke methods dynamically at runtime.
Key Features of Delegates
- Type-Safe: Delegates ensure that the method signature (return type and parameters) matches the delegate’s signature.
- Method References: Delegates can hold references to methods, making them flexible for callbacks and event handling.
- Multicast: A delegate can reference multiple methods and invoke them in a sequence (useful for event handling).
Why Do You Need Delegates in C#?
Delegates in C# are more than just function pointers. They allow you to write flexible, modular, and maintainable code. So, why should you use them in your C# programs? Let’s break it down.
1. Event Handling
Delegates make it simple to trigger an action in response to an event. You can easily assign methods to events and invoke them when needed. This is how event-driven programming works smoothly in C#.
2. Callbacks
Ever needed to pass a method to another method? With delegates, you can do exactly that! They let you pass methods as parameters, which is perfect for implementing callback functions. Imagine needing to execute different actions without knowing what exactly will happen at runtime. That's what delegates help you with.
3. Multicast Delegates
Did you know that delegates can point to multiple methods at once? This allows you to call several methods in a single invocation. It’s really handy when you want the same event to trigger multiple actions, just like how a notification system works with multiple listeners!
Read More: C# Delegates and Plug-in Methods |
4. Code Decoupling
Delegates help keep your code clean by decoupling the method that calls an action from the method that actually performs it. Do you see how this makes your code more maintainable? You can change the action without touching the calling method!
5. Anonymous Methods
Delegates allow you to use anonymous methods or lambda expressions, meaning you don’t need to write full method definitions. Want to write concise and readable code? Delegates give you that power!
Example: Delegate for Event Handling
using System;
public delegate void Notify(); // Delegate declaration
class Program
{
public static event Notify OnNotify; // Event declaration
static void Main()
{
OnNotify += () => { Console.WriteLine("Event Triggered!"); }; // Subscribing to event
TriggerEvent();
}
static void TriggerEvent()
{
OnNotify?.Invoke(); // Event invocation using delegate
}
}
Output
Event Triggered!
Explanation
In this example, the Notify delegate is used to define an event OnNotify. When the event is triggered by calling TriggerEvent(), it invokes the method subscribed to the event, which outputs "Event-Triggered!" to the console.
What Are the Benefits of Delegates?
So, why should you use delegates in C#? Well, delegates offer several powerful benefits that can make your code more flexible, easier to maintain, and more dynamic. Let’s dive into why delegates are so important.
1. Flexibility
With delegates, you can pass methods as parameters. This means you can change what method gets called at runtime, giving you incredible flexibility. Isn’t that a game-changer for dynamic behavior?
2. Event Handling
Delegates are essential for event handling. They allow you to subscribe methods to events and trigger them when needed. This makes your event-driven programming much cleaner and more efficient. Want smoother event handling? Delegates are the way to go.
3. Multicast Capability
Did you know delegates can call multiple methods at once? That’s what we call a multicast delegate. This is perfect when you need to trigger several actions in response to a single event. How cool is that?
4. Code Decoupling
Delegates help you decouple your code. By passing methods as arguments, you don’t need to couple the components of your program tightly. This makes your code more modular and easier to maintain. Wouldn’t you prefer writing clean, maintainable code?
5. Reusability
Delegates allow you to reuse methods across different parts of your application. Since they reference methods with the same signature, you can easily call them wherever needed. More reusable code means more efficient development, right?
In short, delegates bring flexibility, better event handling, multicast capability, code decoupling, and reusability to your C# programs. Doesn’t that sound like something you want in your toolbox?
What Are the Types of Delegates in C#?
Delegates in C# can be classified into several types. Understanding these types helps you choose the right delegate for your needs. Let’s explore them together!
1. Single-Cast Delegate
A single-cast delegate points to a single method. It’s simple and perfect when you only need to invoke one method at a time. Want to trigger one action based on an event or input? This is your go-to delegate.
2. Multi-Cast Delegate
A multi-cast delegate is a type of delegate that can reference multiple methods. When invoked, it calls all the methods in the delegate chain. Isn’t this handy when you want to trigger multiple actions for a single event? It’s great for event handling, where several methods need to be called in response to a single event.
3. Generic Delegate
Generic delegates allow you to define a delegate with a parameterized type. They provide type safety and flexibility, making your code more reusable. Need to work with different data types? Generic delegates have you covered!
4. Anonymous Method Delegate
Anonymous method delegates allow you to define a method inline without giving it a name. This can make your code more concise and easier to read. Why write long method definitions when you can just use an anonymous method?
5. Lambda Expression Delegate
Another form of anonymous method is the lambda expression delegate. It provides a shorthand way to write anonymous methods. Want even shorter, cleaner code? Lambda expressions are the way to go.
In short, C# offers several types of delegates that give you flexibility, efficiency, and cleaner code. Whether you’re dealing with single methods, multiple methods, or anonymous functions, you’ll find the perfect delegate for your needs!
How to Define a Delegate in C#?
There are three essential steps in defining and using delegates in C#. Let’s break them down together, step by step.
Step 1: Declaration of a Delegate
To create a delegate, you need to use the delegate keyword. Here’s how it’s structured:
[attributes] [modifiers] delegate ReturnType Name ([formal-parameters]);
The attributes can be any C# attribute you choose. The Access modifiers can include keywords like new, public, private, protected, or internal. The ReturnType could be any data type, including void or the name of a class. The Name must be a valid C# identifier. You must also include parentheses for method parameters (even if there are none).
Here’s an example of how to define a delegate with no parameters:
public delegate void DelegateExample();
Step 2: Instantiation of Delegate
Once your delegate is defined, you can instantiate it by pointing it to a specific method. Here’s how you do that:
DelegateExample d1 = new DelegateExample(Display);
In this case, d1 is a delegate pointing to the Display method.
Step 3: Invocation of Delegate
Finally, you can invoke the delegate just like a regular method. Here's the invocation:
d1();
When you call d1(), it will execute the method referenced by the delegate (in this case, Display).
In summary, defining and using a delegate in C# involves declaring it, instantiating it with a method, and then invoking it. Simple, right?
1. Single-Cast Delegate Example
A Single-Cast Delegate in C# can reference and invoke only one method. It's simple to use when you need to call a single method at a time.
using System;
public delegate void DisplayMessage(); // Single-cast delegate declaration
class Program
{
static void Main()
{
DisplayMessage messageDelegate = new DisplayMessage(Display); // Instantiating delegate
messageDelegate(); // Invoking delegate
}
static void Display()
{
Console.WriteLine("Hello from Single-Cast Delegate!");
}
}
Output
Hello from Single-Cast Delegate!
Explanation
In this example, a Single-Cast Delegate named DisplayMessage is declared and associated with the Display method. The delegate is then invoked, which calls the Display method and outputs "Hello from Single-Cast Delegate!" to the console.
2. Multi-Cast Delegate Example
A Multi-Cast Delegate can reference and invoke multiple methods. This allows you to call more than one method at a time with a single delegate.
using System;
public delegate void DisplayMessage(); // Multi-cast delegate declaration
class Program
{
static void Main()
{
DisplayMessage messageDelegate = new DisplayMessage(Display1); // Instantiating delegate
messageDelegate += Display2; // Adding another method to the delegate
messageDelegate(); // Invoking delegate, calls both methods
}
static void Display1()
{
Console.WriteLine("Hello from Display1!");
}
static void Display2()
{
Console.WriteLine("Hello from Display2!");
}
}
Output
Hello from Display1!
Hello from Display2!
Explanation
In this example, a Multi-Cast Delegate named DisplayMessage is used to reference two methods, Display1 and Display2. Both methods are invoked when the delegate is called, resulting in two messages being printed to the console.
3. Generic Delegate Example
A Generic Delegate allows you to create a delegate that can work with different data types, making it reusable across multiple methods with varying parameter types.
using System;
public delegate T DisplayMessage(T message); // Generic delegate declaration
class Program
{
static void Main()
{
// Correctly specify the type as 'string' in the delegate instantiation
DisplayMessage messageDelegate = new DisplayMessage(Display); // Instantiating delegate with string type
string result = messageDelegate("Hello from Generic Delegate!"); // Invoking delegate
Console.WriteLine(result);
}
static string Display(string message)
{
return message;
}
}
Output
Hello from Generic Delegate!
Explanation
In this example, a Generic Delegate named DisplayMessage is defined to work with any data type. It is instantiated with a string type, and the delegate returns a string message, which is then printed to the console.
4. Anonymous Method Delegate Example
An Anonymous Method Delegate allows you to define a method inline without explicitly creating a separate method. It’s a quick way to implement simple functionality.
using System;
public delegate void DisplayMessage(); // Delegate declaration
class Program
{
static void Main()
{
DisplayMessage messageDelegate = delegate
{
Console.WriteLine("Hello from Anonymous Method Delegate!");
};
messageDelegate(); // Invoking delegate
}
}
Output
Hello from Anonymous Method Delegate!
Explanation
In this example, an Anonymous Method Delegate is used to define a simple method inline that prints a message to the console. This approach eliminates the need for a separate method definition.
5. Lambda Expression Delegate Example
A Lambda Expression Delegate is a more concise way to define a delegate. It uses a compact syntax to define the method inline, making it ideal for short operations.
using System;
public delegate void DisplayMessage(); // Delegate declaration
class Program
{
static void Main()
{
DisplayMessage messageDelegate = () => { Console.WriteLine("Hello from Lambda Expression Delegate!"); }; // Lambda expression
messageDelegate(); // Invoking delegate
}
}
Output
Hello from Lambda Expression Delegate!
Explanation
In this example, a Lambda Expression Delegate is used to define a delegate with a concise syntax. The lambda expression is invoked to output the message to the console.
How are Delegates Related to Events in C#?
Delegates and Events in C# are closely related, as events are based on delegates. Below are some key points that explain the relationship between delegates and events:
- Delegate as a Foundation: An event in C# is essentially a special type of delegate. While a delegate defines a method signature, an event allows you to associate multiple methods (subscribers) with it, based on that delegate's type.
- Event Declaration: An event is declared using a delegate type. For example, a delegate type
Notify
can be used to declare an event, allowing methods with the same signature to be subscribed to it. - Event Handling: A delegate instance holds references to methods that handle an event. When the event is triggered, the delegate invokes the subscribed methods.
- Single-Cast Delegate: Events can use single-cast delegates, where only one method is invoked when the event is triggered.
- Multi-Cast Delegate: Events support multi-cast delegates, allowing multiple methods to be invoked when the event is raised. This helps implement scenarios where multiple handlers react to a single event.
- Subscription and Unsubscription: With events, methods can be added (subscribed) or removed (unsubscribed) from the event using the
+=
and-=
operators, respectively. This ensures that event handlers can dynamically be managed. - Encapsulation: Events provide encapsulation of the delegate instance, so the delegate cannot be directly invoked outside the event. The event can only be triggered within the class or through special access methods.
- Safety: The event mechanism ensures that delegates are not accidentally invoked from outside the class. This adds a layer of safety, as events are usually invoked only in specific scenarios, such as user actions or system notifications.
In summary, A delegate acts as the foundation for an event, defining the method signature, while the event controls the access to and invocation of the delegate, allowing a safe and organized way to handle multiple method invocations in response to specific actions.
How to Use Events and Delegates in C#?
Once an event is declared in C#, it must be linked to one or more event handlers before it can be triggered. An event handler is simply a method that gets called via a delegate. To associate an event with a delegate, you can use the +=
C# operator.
Here’s an example of associating a delegate with an event:
obj.MyEvent += new MyDelegate(obj.Display);
By default, an event is null if no methods are subscribed to it. Although events are primarily used in Windows-based applications, they can also be utilized in console, web, and other types of applications.
Example 1: Creating a Custom Single-Cast Delegate and Event
This example demonstrates how to create a custom single-cast delegate and event:
using System;
namespace DelegateCustom
{
class Program
{
public delegate void MyDelegate(int a); // Delegate Declaration
public class XX
{
public event MyDelegate MyEvent; // Event Declaration
public void RaiseEvent()
{
// Null check before raising the event
MyEvent?.Invoke(20); // Triggering Event
Console.WriteLine("Event Raised");
}
public void Display(int x)
{
Console.WriteLine("Display Method {0}", x);
}
}
static void Main(string[] args)
{
XX obj = new XX();
obj.MyEvent += new MyDelegate(obj.Display); // Subscribing to event
obj.RaiseEvent();
Console.ReadLine();
}
}
}
Output
Display Method 20
Event Raised
Explanation
In this program, a custom delegate MyDelegate
is used to declare an event MyEvent
. When the event is triggered using the RaiseEvent()
method, the subscribed method Display
gets called, printing the value passed as the parameter (20). After that, the message "Event Raised" is displayed to indicate that the event has been triggered.
Example 2: Creating Custom Multi-Cast Delegates and Events
This example shows how to create a custom multi-cast delegate and event, allowing multiple methods to be triggered by a single event:
using System;
namespace DelegateCustomMulticast
{
class Program
{
public delegate void MyDelegate(int a, int b); // Multi-cast Delegate Declaration
public class XX
{
public event MyDelegate MyEvent; // Event Declaration
public void RaiseEvent(int a, int b)
{
// Null check before raising the event
MyEvent?.Invoke(a, b); // Triggering Event
Console.WriteLine("Event Raised");
}
public void Add(int x, int y)
{
Console.WriteLine("Add Method {0}", x + y);
}
public void Subtract(int x, int y)
{
Console.WriteLine("Subtract Method {0}", x - y);
}
}
static void Main(string[] args)
{
XX obj = new XX();
obj.MyEvent += new MyDelegate(obj.Add); // Subscribing Add method
obj.MyEvent += new MyDelegate(obj.Subtract); // Subscribing Subtract method
obj.RaiseEvent(20, 10); // Invoking the event
Console.ReadLine();
}
}
}
Output
Add Method 30
Subtract Method 10
Event Raised
Explanation
In this program, we define a multi-cast delegate called MyDelegate
that can trigger multiple methods. The event MyEvent
is associated with two methods: Add
and Subtract
. Both methods are invoked when the event is raised by the RaiseEvent()
method, performing addition and subtraction of the two values passed as arguments (20 and 10), respectively. Finally, the message "Event Raised" is displayed to indicate that the event has been triggered.
Read More: C# 12 Developer Roadmap |
Summary
Delegates in C# are powerful tools that provide a way to reference methods and define callback functions. Delegates enable you to implement event handling and multi-casting and provide greater flexibility in the execution of methods. They are often used in scenarios like event-driven programming, asynchronous operations, and implementing the observer design pattern. By mastering delegates, you can significantly enhance your C# programming skills and improve the structure of your code. Want to dive deeper into C# and improve your programming expertise? Check out the Full-Stack .NET Developer Certification Training Course for hands-on practice and real-world projects.
Exclusive Free Courses
Scholarhat offers amazing free courses to help you build job-ready skills and boost your career. Check them out below:
- Free ASP.NET Core Course – Learn the basics of building web applications with ASP.NET Core.
- Free C# Course With Certificate – Dive deep into building C# Concepts and more.
Test Your Knowledge of C# Delegates!
Q 1: What is a delegate in C#?
- (a) A type-safe function pointer
- (b) A type of collection
- (c) A way to store objects
- (d) A base class for events
Q 2: Which of the following is true about delegates in C#?
- (a) A delegate can only point to a static method
- (b) A delegate can point to multiple methods
- (c) Delegates cannot be used with events
- (d) A delegate cannot return a value
Q 3: What is the difference between a delegate and an event in C#?
- (a) Events cannot have multiple handlers, but delegates can
- (b) Delegates are used to declare events
- (c) Events are a type of delegate that can be subscribed to
- (d) Events can only be invoked within the class that declares them
Q 4: How do you declare a delegate in C#?
- (a) delegate void MyDelegate();
- (b) MyDelegate() delegate;
- (c) delegate MyDelegate();
- (d) void delegate MyDelegate();
Q 5: How do you invoke a delegate in C#?
- (a) delegate.Invoke();
- (b) delegate();
- (c) Invoke(delegate);
- (d) delegate.Execute();
FAQs
Take our Csharp skill challenge to evaluate yourself!
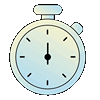
In less than 5 minutes, with our skill challenge, you can identify your knowledge gaps and strengths in a given skill.