25
AprUnderstanding C# Interface
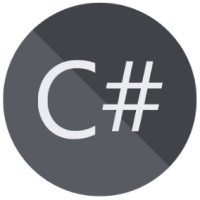
C# Programming For Beginners Free Course
Interface: An Overview
An interface acts as a contract between itself and any class or structure that implements it. It means a class that implements an interface is bound to implement all its members. An interface has only a member’s declaration or signature and implicitly every member of an interface is public and abstract. In this C# Tutorial, we will explore more about Interfaces which will include c# interface with real-life examples, when to use interface with examples, design guidelines for Interface, and disadvantages of interfaces.
What is the interface in c#?
- An interface looks like a class but It has no implementation.
- It only contains declarations of events, indexers, methods, and/or properties.
- The reason behind this is that interfaces are inherited by structs and classes, which must provide an implementation for each interface member declared.
- The interface is a completely abstract class.
- Which only contain abstract methods and properties.
Syntax of interface
// interface
interface Employee
{
void employeename(); // interface method (does not have a body)
void salary(); // interface method (does not have a body)
}
Real-World Example of Interface
Let's elaborate on the real-world example in C# Compiler.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text.RegularExpressions;
namespace HelloWorld
{
interface ICompany
{
void companyname(); // interface method (does not have a body)
}
class Employee : ICompany
{
public void companyname()
{
Console.WriteLine("Scholarhat");
}
}
class Program
{
static void Main(string[] args)
{
Employee emp = new Employee(); // Create a Employee object
emp.companyname();
}
}
}
Output
Scholarhat
Read More - C# Interview Questions And Answers
Implementing multiple interfaces:
using System;
namespace MyFamily
{
interface ImotherInterface
{
void Mother(); // interface method
}
interface IFatherInterface
{
void Father(); // interface method
}
// Implement multiple interfaces
class ChildClass : ImotherInterface,IFatherInterface
{
public void Mother()
{
Console.WriteLine("Mother's DNA");
}
public void Father()
{
Console.WriteLine("Father's DNA");
}
}
class Program
{
static void Main(string[] args)
{
ChildClass child = new ChildClass();
child.Mother();
child.Father();
}
}
}
Output:
Mother's DNA
Father's DNA
Features of Interface
An interface doesn't provide inheritance like a class or abstract class but it only declares members which an implementing class needs to be implemented.
It cannot be instantiated but it can be referenced by the class object which implements it. Also, Interface reference works just like object reference and behaves like an object.
IStore IObjStore = new Document(); ICompress IObjCompress = new Document();
It contains only properties, indexers, methods, delegates, and event signatures.
It cannot contain constant members, constructors, instance variables, destructors, static members, or nested interfaces.
Members of an interface cannot have any access modifiers even the public.
Implicitly, every member of an interface is public and abstract. Also, you are not allowed to specify the members of an interface public and abstract or virtual.
An interface can be inherited from one or more interfaces.
An interface can extend another interface.
A class or struct can implement more than one interface.
A class that implements an interface can mark any method of the interface as virtual and this method can be overridden by derived classes.
Implementing multiple interfaces by a class sometimes results in a conflict between member signatures. You can resolve such conflicts by explicitly implementing an interface member.
It is a good practice to start all interface names with a capital “I” letter.
Common Design Guidelines for Interface
Keep your interfaces focused on the problem you are trying to solve and keep related tasks (methods) in an interface. Interfaces that have multiple unrelated tasks tend to be very difficult to implement in a class. Split up interfaces that contain unrelated functionality.
Make sure your interface does not contain too many methods. Since too many methods make implementing the interface difficult as the implementing class has to implement each and every method in the interface.
Don't make interfaces for specific functionality. An interface should define the common functionality that can be implemented by the classes of different modules or subsystems.
When to use interfaces?
Need to provide common functionality to unrelated classes.
Need to group objects based on common behaviors.
Need to introduce polymorphic behavior to classes since a class can implement more than one interface.
Need to provide a more abstract view of a model which is unchangeable.
Need to create loosely coupled components, easily maintainable and pluggable components (like log4net framework for logging) because the implementation of an interface is separated from itself.
Disadvantage of Interface
The main issue with an interface is that when you add a new member to it, then you must implement those members within all of the classes that implement that interface.
Interfaces are slow as these require extra in-direction to find the corresponding methods in the actual class.
Conclusion:
I hope, now you have got everything about Interface. I would like to have feedback from my blog readers. Your valuable feedback, questions, or comments about this article are always welcome. Also, Consider our C# Programming Course for a better understanding of all C# concepts.
FAQs
Q1. What are the most important interfaces in C#?
- IComparable.
- IDisposable.
- IFormattable.
- IEnumerable.
- IList.
Q2. When would you use an interface C#?
Q3. What is difference between interface and abstract class in C#?
Take our free csharp skill challenge to evaluate your skill
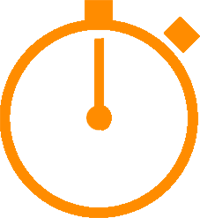
In less than 5 minutes, with our skill challenge, you can identify your knowledge gaps and strengths in a given skill.