Angular 6 Tutorial - Learn Angular 6 in 60 Minutes
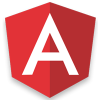
Angular Course
Angular is a JavaScript framework for building apps and web applications in HTML, JavaScript, and TypeScript which is a superset of JavaScript. Angular provides built-in features for the HTTP services, animation, and materials which in turn provide features of auto-complete, toolbar, navigation, menus, etc.
Angular Tutorial
Angular is a JavaScript framework for building apps and web applications in HTML, JavaScript, and TypeScript which is a superset of JavaScript. Angular provides built-in features for the HTTP services, animation, and materials which in turn provide features of auto-complete, toolbar, navigation, menus, etc. The Angular Code is written in TypeSript but again compiles into JavaScript and displays the same thing in the browser.
Know About Angular:
Angular is a JavaScript Framework that can make you able to generate reactive Single Page Applications (SPAs). This is one of the leading front-end development frameworks which is regularly updated by the team of Angular of Google. Angular is based on the components where so many components form a tree structure with child and parent components.
Angular solves most of the challenges faced while developing single-page applications (SPA), cross-platform, performance-centric applications. It's fully extensible and works very well with other libraries such as React or VueJs using one of the features called Angular Elements. Exploring an Angular Certification Course can provide in-depth insights into these capabilities, empowering individuals to harness Angular's potential for building robust, scalable, and interoperable applications.
Prerequisite:
Before starting to learn and gain depth knowledge in Angular, you must have the basic knowledge of AngularJS, Visual Studio Code IdE, JavaScript, and concepts of any server-side programming language.
How to Install Angular:
The easiest and quickest way to start the Angular app is through the Angular CLI(Command Line Interface). To install it, you required either the node package manager or the yarn package manager. To check, whether or not you have npm in your console or command the yarn package manager line, you need the yarn package manager to type:
>npm –v
If the above code goes unrecognized or does not show any version number, you have to install NodeJS. Once NoeJS has been installed, reload the command line or console that you will have access to NPM. Now, you can use the NPM to install the Angular CLI. To install it write:
>npm install –g @angular/cli
After installing if you run ng –v, it will show you the version number which maybe 6.0.7. So, once the CLI is installed, the developer can use it to start to install a new Angular project.
>ng new ng6 – proj –style=scss –routing
On the above code what we can understand is mentioned below:
ng – In this way, we can call the Angular CLI.
new – It is one of the many commands that the developer can issue to the CLI.
ng6 –
proj: It is the name for which we are calling our project. The CLI can create the folder with this name, based on where you are running the command.
Optional Flags: There are two optional flags that I decided to add. First, we have to tell the CLI to generate the project that has SaSS enabled (by default, CSS is being used if it will not be specified), and then routing is added because we want a project which has different page URL’s and adding this flag will help to create a routing file for us.
So, to check all the available commands, you can run ng at the command line. Once the CLI has generated the new project, you can jump into it:
>cd ng6-proj
Now, if you want to use Visual Studio Code, you can type the code to launch the code editor, and to serve it to the browser, you can run:
>ng serve -o
The –o flag will let the CLI launch the browser with the project. Now, the developer can watch the Angular project as you code it, it will then automatically live to reload.
Angular Project Structure:
While you view the folder and file structure of the Angular app, it would look like something almost similar to the below code:
>e2e >node_modules >src >app ….a bunch of files …a bunch of files
You as a developer may be going to spend most of your time working within the /src/app folder. This is a place where components and services are stored.
Now, in the /src/ folder itself, you can see an index.html (which is the entry point of the apps) and a style.css file which is where the global CSS rulesets have been placed.
The /src/assets folder is the place where the developer can place any assets like the graphics.
Another /dist/ folder can also be generated when you build the project.
Angular Module File:
Before going to tackle anything, it is always important to look at the /src/app/app.module.ts file. The .ts extension here represents the TypeScript which is used by Angular. TypeScript provides strong type checking on JavaSCript.
Angular Module mainly mentions the place where the developer can group the components, pipes, directives, and services that are related to the application. Now, when you are developing the website, the header, footer, center, left and right sections become part of the module. So, to describe the module, we can use the NgModule. When you create a new project using the Angular – CLI command, where the ngmodule has been created by default in the app.module.ts file. Now, the app.module file may look like the below:
import { BrowserModule } from '@angular/platform-browser'; import { NgModule } from '@angular/core'; import { AppRoutingModule } from './app-routing.module'; import { AppComponent } from './app.component' @NgModule({ declarations: [ AppComponent ], imports: [ BrowserModule, AppRoutingModule ], providers: [], bootstrap: [AppComponent] }) export class AppModule { }
So, whenever you will use the CLI to create services and components, it will then automatically update the file to import and add them to the @NgModule decorator. In Angular, the Components are added to the declarations array, and services are added as providers. The developers are also adding different imports to the imports array. Even, when you want to add animation, you can add them here as well.
Angular Components:
Most of the important part of the development with Angular is done in the components. Components are main classes that can be able to interact with the .html file of the component which gets displayed on the browser. The file structure has the app component and it consists of the following app files:
- component.html
- component.css
- component.ts
- component.spec.ts
- module.ts
Angular is a single-page application (SPA) framework, and a view is made of one or more components. An Angular component represents a portion of a view and Generally, an interactive web page is made of HTML, CSS, and JavaScript and the angular component is no different as it follows the same approach where component represents business logic, template represents HTML and CSS file consist stylesheet.
Angular Routing:
In Angular, routing plays a crucial role since it is essentially used to create Single-page Applications (SPA). These applications are loaded just once in a while compared to server-side applications, and the new changes are added dynamically based on the user interactivity.
Applications like Google, Facebook, and Gmail are a few examples of Single-page Applications (SPA) and the best advantage of Single-page Applications (SPA) is that it provides an excellent user experience and we don’t have to wait for pages to load for multiple minutes to interact with UI, and by extension, this feature makes the Single-page applications fast and gives a best possible performance over other programming platforms.
Now, if you want to work the routing, then you have to visit the /src/app/app-routing.module.ts file. With the code, it will look like this:
import { NgModule } from '@angular/core'; import { Routes, RouterModule } from '@angular/router'; const routes: Routes = []; @NgModule({ imports: [RouterModule.forRoot(routes)], exports: [RouterModule] }) export class AppRoutingModule { }
After the addition of that code, you have to import the components at the top, and then you need to add them to the Routes. So, to import the component, the below-mentioned code needs to be added:
import { NgModule } from '@angular/core'; import { Routes, RouterModule } from '@angular/router'; import { UsersComponent } from './users/users.component'; import { DetailsComponent } from './details/details.component'; import { PostsComponent } from './posts/posts.component'; const routes: Routes = [ { path: '', component: UsersComponent }, { path: 'details/:id', component: DetailsComponent }, { path: 'posts', component: PostsComponent }, ];
Here, in the above code, three components have been defined, and after that three objects are in the Routes array.
In the above-mentioned code, the first object identifies that the UserComponent will be the default component that loads on the root path. We can leave the path value blank for this. The next Route is specifying the User details section. Here, a Wild car named Id has been mentioned so that you can use this to fetch the value from the router to retrieve the correct user details. Another route for a component and path is termed as posts. After saving the file, the browser will show: “User Works”.
Angular Services
If you are fetching a list of users from the public API, then the User Components will work. For this, you need to use the Angular CLI to generate a Service for all. Angular Service is very important and useful to place the code which is reusable throughout different components of the app. So, in the console, write:
>ng generate service data
After opening the new service file - /src/app/data.service.ts, write:
import { Injectable } from '@angular/core'; @Injectable({ providedIn: 'root' }) export class DataService { constructor() { } }
It will almost look similar to the regular component. You can define the imports at the top and your methods and properties in the class that is exported.
Angular Data Binding
Data binding is not only available in Angular6 but it has been started from Angular JS, Angular 2, and 4 also. The developers are using curly braces for the data binding {{ }} and this process is termed interpolation. The variable in the app.component.html file is mainly termed as {{title}} and the value of the title is initialized in the app.component.ts file and it has been displayed in the app.component.html. Now, if you want to create a dropdown of months in the browser, you have to create the array of months in the app.component.ts as follows:
import { Component } from '@angular/core'; @Component({ selector: 'app-root', templateUrl: './app.component.html', styleUrls: ['./app.component.css'] }) export class AppComponent { title = 'Angular 6 Project!'; // declared array of months. months = ["January", "February", "March", "April", "May", "June", "July", "August", "September", "October", "November", "December"]; }
Now, if you want the array of the months which has been shown in the above code is to be displayed on the dropdown section of the browser, the following line of code need to write:
<div style="text-align:center"> <h1> Welcome to {{title}}. </h1> </div> <div> Months : <select> <option *ngFor = "let I of months">{{i}}</option> </select> </div>
Here, in the above code, a normal select tag has been used with the option. Again the option, a for loop is used to iterate over the months’ array which in turn will create the option tag with the value in the months. The syntax ‘for’ is used in Angular as *ngFor = “let I of months” and to know the value of the months, we have to display it in {{ I }}.
The above-mentioned two curly brackets help in data binding. You can declare the variables in the app.component.ts file and the same can be easily replaced by using the curly brackets.
Event Binding
When any user interacts with an application in the form of a keyboard movement, a mouse click, or a mouseover, it will then generate an event. Such events are required to handle the performance of some types of actions. At that moment, event binding of Angular comes.
In Angular, event binding is used to handle the various events raised by the user actions like button click, mouse interactivity, keystrokes, etc. When the DOM event happens at an element based on the different behavior (i.e. click, key down, key up), it calls the specified method in the particular component.
Using Event Binding we can bind data from DOM to the component and hence can use that data for further purposes.
Angular Templates
Angular uses the <ng-template> as the tag is almost the same as the Angular 4 tag instead of <template> that is used in Angular 2. The template has been changed in Angular 4 from <template> to <ng-template> because there is a name <template> in the HTML tag which can be a conflict with the Angular template name.
Angular directives
Directives in Angular are TypeScript classes that are declared with the decorator @Directive and these are the Document Object Model (DOM) instruction sets that decide how the logic implementation can be done, in other words, directives are designed to manipulate the DOM based on the various set of instruction/conditions. Exploring dedicated Angular JS Training can provide a comprehensive understanding of directives and their utilization, empowering individuals to effectively manipulate the DOM and enhance the functionality of Angular applications.
The directive in Angular is a JS Class that is called @directive. We have mainly 3 directives in Angular as mentioned below:
- Component Directives
- Structural Directives
- Attribute Directives
What are the new features in Angular?
Updated Component Development Kit (CDK): It supports creating custom UI elements without the requirement of an angular material library. It also supports responsive web design layouts and overlay packages and overlay packages to create pop-ups.
Updated Angular Command Line Interface (CLI): In Angular, new commands have been added like the ng-update which was migrated from its previous version to the current version. Ng-add is quickly adding the application features to make any application progressive web apps.
The most recent features include the Ivy Renderer engine, Angular CLI prompts and improved logging & reporting, comprehensive progressive web apps, Angular Universal, Angular elements, and many more.
Usage of RxJs, a reactive JS library
Updated Angular Material: So many new Tree components have been added along with mat-tree, ck-tree, a styled version, an unstyled version to represent the hierarchical structure like Tree.
Angular Element: Angular allow the Angular components to be published as Web Components which can then also be used in any HTML Page. Even, native custom elements can also be created by using the Angular Element package.
Tree Shaking on Services: Tree Shaking in Angular can be applied to services to remove any dead code.
Multiple Validators: It will allow the validators to apply to the form builder.
Take our free angular skill challenge to evaluate your skill
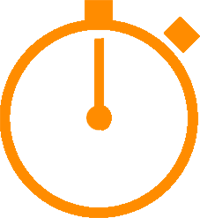
In less than 5 minutes, with our skill challenge, you can identify your knowledge gaps and strengths in a given skill.