Constructor Chaining in Java
Constructor Chaining in Java: An Overview
Constructor Chaining in Java plays a very important role in a Java Program, especially when we are dealing with multiple constructors in a class. In this Java Tutorial, we'll explore What is Constructor Chaining in Java?, What is the use of Constructor Chaining?, Constructor Chaining in Java with Examples. To enhance your knowledge in Java, we advice you to enroll in our Java Certification Program.
What is Constructor Chaining in Java?
We assume you already know what are Constructors in Java. They are the special methods which help in initializing newly created objects. One of the concepts related to constructors is Constructor Chaining.
- Constructor Chaining is a mechanism in Java where one constructor calls another constructor within the same class or in its superclass.
- It works like a chain, where one constructor leads to another and the execution of multiple constructors occurs in a sequence.
- To chain constructors within the same class, we use this() keyword and super() to call constructor from the parent class.
Read More - Advanced Java Interview Questions And Answers
Read More - Java Multithreading Interview Questions
Types of Constructor Chaining
- Chaining within the same class this type of constructor chaining in Java, chaining occurs within the same class, that is, a constructor calls another constructor of the same class to which it belongs.
- Chaining with Superclass Constructors- With the help of Inheritance, a subclass can inherit from a superclass. Constructor Chaining with superclass constructors is when a constructor in a subclass calls a constructor from its superclass using the ‘super()’ keyword. This ensures proper execution of initialization tasks in both superclass and subclass when an object is created in the subclass.
Syntax
public class MyClass {
// Constructor with parameters
public MyClass(int param1, String param2) {
// Some initialization or processing
// Call another constructor within the same class
this(param1);
// Additional initialization or processing
}
// Constructor with a different set of parameters
public MyClass(int param1) {
// Some initialization or processing
// Additional initialization or processing
}
}
Syntax
public class Subclass extends Superclass {
// Constructor with parameters
public Subclass(int param1, String param2) {
// Call superclass constructor with parameters
super(param1, param2);
// Additional initialization or processing
}
}
How Constructor Chaining Works in Java?
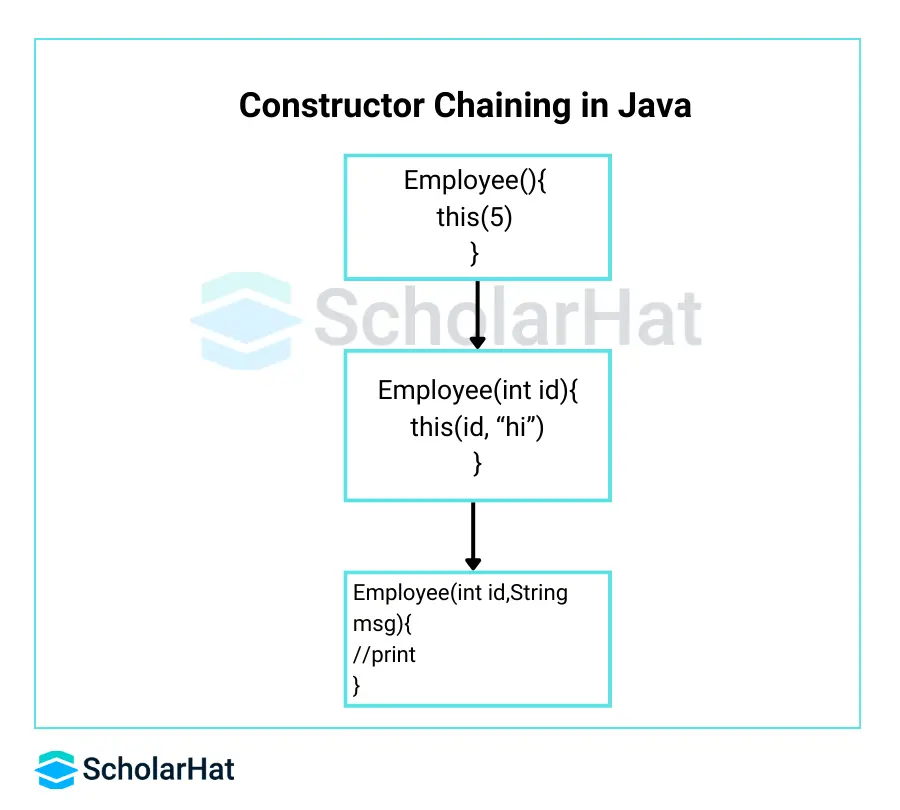
Example on our Java Compiler
public class MyClass {
private int value1;
private String value2;
// Constructor with parameters
public MyClass(int value1, String value2) {
// Call another constructor within the same class
this(value1);
// Some initialization or processing
this.value2 = value2;
// Additional initialization or processing
System.out.println("Constructor with int and String parameters called.");
}
// Constructor with a different set of parameters
public MyClass(int value1) {
// Some initialization or processing
this.value1 = value1;
// Additional initialization or processing
System.out.println("Constructor with int parameter called.");
}
public void displayValues() {
System.out.println("Value1: " + value1);
System.out.println("Value2: " + value2);
}
public static void main(String[] args) {
// Creating an instance of MyClass
MyClass obj = new MyClass(10, "Hello");
// Displaying values
obj.displayValues();
}
}
In the above example,
- There are two constructors in the class MyClass, one has two parameters and another only one.
- The constructor that has two parameters, int and string, calls the other constructor that has only one parameter, int using the this() keyword.
Output
Constructor with int parameter called.
Constructor with int and String parameters called.
Value1: 10
Value2: Hello
Chaining Constructors in Superclasses
class Vehicle {
private String brand;
private String model;
// Constructor with parameters
public Vehicle(String brand, String model) {
this.brand = brand;
this.model = model;
System.out.println("Vehicle constructor called with brand: " + brand + ", model: " + model);
}
}
class Car extends Vehicle {
private int year;
// Constructor with parameters
public Car(String brand, String model, int year) {
super(brand, model); // Call superclass constructor with parameters
this.year = year;
System.out.println("Car constructor called with brand: " + brand + ", model: " + model + ", year: " + year);
}
}
public class Main {
public static void main(String[] args) {
// Creating an instance of Car
Car car = new Car("Toyota", "Corolla", 2020);
}
}
In the above example,
- There is a superclass Vehicle, which has attributes as brandand model.
- It has a subclass Car, with another attribute year.
- Using the super() keyword, the constructor of the Car class calls the constructor of Vehicle class.
- In the main()method, when an instance of Caris created, both the constructors of Car and Vehicle are called.
Output
Vehicle constructor called with brand: Toyota, model: Corolla
Car constructor called with brand: Toyota, model: Corolla, year: 2020
Implicit Super Constructor Call
Example:
class Superclass {
int num;
// Superclass constructor
public Superclass() {
System.out.println("Superclass constructor called.");
num = 10;
}
}
class Subclass extends Superclass {
// Subclass constructor
public Subclass() {
// Call to superclass constructor
super();
System.out.println("Subclass constructor called.");
}
// Method to display the value of num
public void display() {
System.out.println("Value of num in superclass: " + num);
}
}
public class Main {
public static void main(String[] args) {
// Creating an object of Subclass
Subclass obj = new Subclass();
// Calling display method
obj.display();
}
}
Explanation:
- We have two classes here, ‘Superclass’ and ‘Subclass’ in our Java Online Editor.
- Subclass inherits from Superclass.
- Superclass has a default constructor that initializes the variable ‘num’ to 10.
- Subclass does not define any constructors explicitly.
- When an object of Subclass is created (‘Subclass obj = new Subclass();’), the constructor of Superclass is implicitly called before the constructor of Subclass.
- The Java compiler calls the superclass constructor implicitly by itself and ‘Superclass’ execution occurs first, before the execution of the ‘Subclass’.
Output
Superclass constructor called.
Subclass constructor called.
Value of num in superclass: 10
Benefits of Constructor Chaining
- Code Reusability-Constructor Chaining allows you to reuse code when there are multiple constructors by calling one constructor from another within the same class or its superclass instead of duplicating it.
- Organization- When we chain constructors, we get a more logically organized code. The code becomes more readable.
- Flexibility- Constructor Chaining allows you to define multiple constructors with different sets of parameters or initialization tasks making the process of object initialization more flexible. Moreover, constructor can be chosen based on specific requirements, making the interface more versatile.
- Initialization Order- When one constructor calls another, it makes sure that any common or superclass initialization tasks are executed before the specific tasks of the current constructor, ensuring that initialization tasks are performed in the correct order.
Rules of Constructor Chaining
- First Statement- The first statement in the constructor must be the call to another constructor, that is, ‘this()’ or ‘super()’.
- One Call constructor can only call one another constructor, be it from the same class or its superclass.
- No Circular Chains- Constructor chaining must be done in a way that it should not create a circular chain, where one constructor indirectly calls itself.
Using the init() method for Constructor Chaining
Example
class MyClass {
private int value1;
private String value2;
// Constructor with parameters
public MyClass(int value1, String value2) {
// Perform some initialization or processing
init(value1, value2);
System.out.println("Constructor with int and String parameters called.");
}
// Constructor with a different set of parameters
public MyClass(int value1) {
// Perform some initialization or processing
init(value1, "Default");
System.out.println("Constructor with int parameter called.");
}
// Private initialization method
private void init(int value1, String value2) {
this.value1 = value1;
this.value2 = value2;
// Additional initialization or processing can be done here
}
public void displayValues() {
System.out.println("Value1: " + value1);
System.out.println("Value2: " + value2);
}
public static void main(String[] args) {
// Creating an instance of MyClass
MyClass obj1 = new MyClass(10, "Hello");
MyClass obj2 = new MyClass(20);
// Displaying values
obj1.displayValues();
obj2.displayValues();
}
}
In the above example,
- We have used the private init() method for initializing the instance variables.
- There are two constructors in MyClass and both of them call the init method with parameters needed.
Output
Constructor with int and String parameters called.
Constructor with int parameter called.
Value1: 10
Value2: Hello
Value1: 20
Value2: Default
Why do we need Constructor Chaining?
Summary
FAQs
Q1. When do we use constructor chaining in Java?
Q2. How to call one constructor from another constructor in Java?
- Use ‘this()’ keyword with appropriate arguments to call another constructor in the same class.
- Ensure that the call to another constructor is the first statement in the constructor where it is called.