11
JulParameterized Constructor in Java
Parameterized Constructor in Java: An Overview
Parameterized Constructors in Java are those kind of constructors that can take one or more parameters during the process of object creation. While we have constructors that are used to set initial values for the attributes of the object, parameterized constructors advances it by allowing the passing of arguments to the constructor when the object is created. In this Java tutorial, we'll learn about them in detail. To enhance your knowledge in Java, consider enrolling in our Java Full Stack Developer Course.
Constructors in Java
First of all, let us recall what are constructors in Java. Constructors are those special type of methods that are used to initialize objects of a class. Moreover, they have the same name as the class name and are invoked whenever an object of that specific class is created. Learn more about Constructors in Java and its types.
Read More - Top Java Interview Questions And Answers
Read More - Top Java Multithreading Interview Questions
What is a Parameterized Constructor
A Parameterized Constructor is a type of constructor that take one or more parameters when the object is created and also allows the developer to initialize the attributes of the object by providing values of their own interest. That is how parameterized constructors in Java give greater flexibility while initializing objects as the initial state of an object can be easily customized based on the specific values provided at the time of creation.
Syntax of Parameterized Constructor
The syntax of the Parameterized constructor in Java is as follows:
public class MyClass {
// Declare instance variables
int number;
String text;
// Parameterized Constructor
public MyClass(int num, String str) {
// Initialize instance variables using constructor parameters
number = num;
text = str;
}
}
Here, 'MyClass' is a class with a parameterized constructor that takes two parameters that are- an integer 'num' and a string 'str'. Within the constructor, these parameters are used to initialize the instance variables 'number' and 'text' respectively.
Example Program:
public class Main {
public static void main(String[] args) {
// Create an object of MyClass using the parameterized constructor
MyClass obj = new MyClass(10, "Hello");
// Access object attributes
System.out.println("Number: " + obj.number);
System.out.println("Text: " + obj.text);
}
}
In the above example, while we create the object of 'MyClass', we pass '10' as the value of 'num' and '"Hello"' as the value of 'str'. These values are further used to initialize the attributes, 'number' and 'text', of the object.
Output
Number: 10
Text: Hello
Why Parameterized Constructors are used?
Parameterized Constructors play a vital role in the initialization and customization of objects in Java programs. Some of its advantages are listed below:
- Customization- With the help of parameterized constructors, you can easily customize the values while initializing objects based on the arguments provided.
- Code Reusability- Parameterized constructors let you reuse the same constructor logic if you want to create an object with a different initial state.
- Encapsulation and Data Integrity- They help maintain data integrity and prevent unauthorized access by encapsulating the initialization logic within the constructor promoting the OOPs concepts in Java.
- Constructor Overloading- Parameterized constructors also support constructor overloading and lets you define multiple constructors with different parameter lists within the same class.
- Clearer Object Creation- The setting of initial values of object attributes is more clear and concise at the moment of object creation, simplifying the code and making it more readable.
Default Constructor vs Parameterized Constructor
Default Constructor | Parameterized Constructor |
A default constructor is a type of constructor which is created by the compiler itself when no constructor is defined in the class. | A parameterized constructor is provided by the developer with one or more than one parameters. |
Object attributes are initialized with default values. | Object attributes are initialized with specific values provided by the user. |
Constructor Overloading
Example
public class Rectangle {
private int length;
private int width;
// Constructor with no parameters
public Rectangle() {
length = 0;
width = 0;
}
// Constructor with one parameter (for square)
public Rectangle(int side) {
length = side;
width = side;
}
// Constructor with two parameters (for rectangle)
public Rectangle(int length, int width) {
this.length = length;
this.width = width;
}
// Method to calculate area
public int calculateArea() {
return length * width;
}
public static void main(String[] args) {
Rectangle square = new Rectangle(5); // Creating a square
System.out.println("Area of the square: " + square.calculateArea());
Rectangle rectangle = new Rectangle(4, 6); // Creating a rectangle
System.out.println("Area of the rectangle: " + rectangle.calculateArea());
}
}
Here, we have a class 'Rectangle' which contains three constructors:
- one constructor without parameters, that has initialized 'length' and 'width' to 0.
- one has a parameter, that will create a square and set the value of 'length' and 'width' to same value.
- another one has two parameters, that will create a rectangle and set the 'length' and 'width' to different values.
Now, the constructor will be called based on the parameters given to it.
Output
Area of the square: 25
Area of the rectangle: 24
Summary
FAQs
Take our Java skill challenge to evaluate yourself!
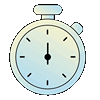
In less than 5 minutes, with our skill challenge, you can identify your knowledge gaps and strengths in a given skill.