14
JunJava Interview Questions for Freshers, 3, 5, and 10 Years Experience
Java Interview Questions for 10 Years' Experience are crucial for senior developers aiming for leadership roles in software development. With 10 years of experience, you are expected to have deep knowledge of Java Core, Multithreading, Collections, JVM internals, Design Patterns, Microservices, and Spring Boot. Employers also look for expertise in performance optimization, system design, and cloud-based architectures.
In this Java tutorial, we provide a list of advanced Java interview questions tailored for professionals with a decade of experience. Whether you're preparing for a technical lead, architect, or senior developer role, this guide will help you showcase your expertise and ace your interview.
Basic Java Interview Questions for Freshers
If you are a fresher preparing for a Java interview, these basic questions will help you understand fundamental concepts like variables, data types, OOP principles, and exception handling. They will also boost your confidence in answering Java-related questions effectively.
- Differentiate between Java and C++.
The following differences are there:
- C++ is only a compiled language, whereas Java is a compiled and interpreted language.
- Java programs are machine-independent, whereas a C++ program can run only on the machine on which it is compiled.
- C++ allows users to use pointers in the program. Whereas it's not the case with Java. Java internally uses pointers.
- C++ supports the concept of multiple inheritances, while Java doesn't support this. It can be achieved by using interfaces in Java.
- Is Java a complete object-oriented programming language?
- Everything in Java is under the classes. And we can access that by creating the objects. This makes it an object-oriented language.
- But it has the support of primitive data types like int, float, char, boolean, double, etc. which is not a feature of object-oriented languages.
- Therefore, Java is not a pure object-oriented programming language because the primitive data types it supports don't directly belong to the Integer classes.
- What is JVM?
JVM stands for Java Virtual Machine. It is a Java interpreter. It is responsible for loading, verifying, and executing the bytecode created in Java. Its implementation is known as JRE. Although it is platform dependent, which means the software of JVM is different for different Operating Systems, it plays a vital role in making the Java platform Independent.
- What do you mean by JIT?
JIT stands for Just-in-Time compiler and is a part of JRE(Java Runtime Environment). It is used for better performance of the Java applications during run-time. The step-by-step working process of JIT is mentioned below:
- Source code is compiled with Javac compiler to form bytecode
- Bytecode is further passed on to JVM. The .class files are loaded at run time by JVM and with the help of an interpreter, these are converted to machine-understandable code.
- JIT is a part of JVM. When the JIT compiler is enabled, the JVM analyzes the method calls in the .class files and compiles them to get more efficient and native machine code at run time. It also ensures that the prioritized method calls are optimized.
- The JVM then executes the optimized code directly instead of interpreting it again, which increases the performance and speed of the execution.
- What gives Java its 'write once and run anywhere' nature?
It is the bytecode. Java compiler converts the Java programs into the .class file known as the Byte Code, which is the intermediate language between source code and machine code. This bytecode is not platform-specific and can be executed on any machine.
- What is a ClassLoader?
Java Classloader is the program of JRE (Java Runtime Environment). The task of ClassLoader is to dynamically load the required Java classes and interfaces to the JVM during the execution of the bytecode or created .class file. Because of classloaders, the Java run time system does not need to know about files and file systems.
- Explain public static void main(String args[]) in Java.
- Public: the public is the access modifier responsible for mentioning who can access the element or the method and what the limit is. It is responsible for making the main function globally available. It is made public so that JVM can invoke it from outside the class, as it is not present in the current class.
- Static: It is a keyword with which you can use the element without initiating the class to avoid the unnecessary allocation of the memory.
- Void: void is a keyword used to specify that a method doesn’t return anything. We use void with the main function because it doesn’t return anything.
- Main: main helps JVM to identify that the declared function is the main function.
- String args[]: It stores Java command-line arguments and is an array of type java. lang.String class.
- Can the main method be overloaded?
Yes, the main method can be overloaded. We can create as many overloaded main methods as we want. However, JVM has a predefined calling method that JVM will only call the main method with the definition of
public static void main(string[] args)
Example
We'll see the demonstration in the Java Compiler
class Main {
public static void main(String[] args) {
System.out.println("This is the main method");
}
public static void main(int[] args) {
System.out.println("Overloaded integer array main method");
}
public static void main(char[] args) {
System.out.println("Overloaded character array main method");
}
public static void main(double[] args) {
System.out.println("Overloaded double array main method");
}
public static void main(float args) {
System.out.println("Overloaded float main method");
}
}
Output
This is the main method
- What are the different data types in Java?
The data types in Java are categorized into two categories, as mentioned below:
- Primitive Data Type
- Non-primitive data Type or Object Data type
- Primitive Data Type: Primitive data are single values with no special capabilities. There are 8 primitive data types in Java:
- boolean: stores value true or false
- byte: stores an 8-bit signed two’s complement integer
- char: stores a single 16-bit Unicode character
- short: stores a 16-bit signed two’s complement integer
- int: stores a 32-bit signed two’s complement integer
- long: stores a 64-bit two’s complement integer
- float: stores a single-precision 32-bit IEEE 754 floating-point
- double: stores a double-precision 64-bit IEEE 754 floating-point
- Non-Primitive Data Type: They are reference data types that contain a memory address of the variable’s values because it is not able to store the values in the memory directly. Types of Non-Primitive data types are:
- String
- Array
- Class
- Object
- Interface
Data Types in Java with Examples | Primitive and Non-Primitive Data Types in Java |
- How many types of operators are there in Java?
There are multiple types of operators in Java. They are as follows:
- Arithmetic operators in java
- Relational operators in java
- Logical operators
- Assignment operators
- Unary operators
- Bitwise operators
Operators in Java | Arithmetic, Relational, Logical, and More |
- Differentiate between
equals()
method and equality operator ==
in Java
equals()
method and equality operator ==
in Javaequals() | equality operator (==) |
This is a method defined in the Object class. | It is a binary operator in Java. |
As the .equals() method is present in the Object class, we can override our custom .equals() method in the custom class, for object comparison. | It cannot be modified. They always compare the HashCode. |
This method is used for checking the equality of contents between two objects as per the specified business logic. | This operator is used for comparing addresses (or references), i.e., checking if both objects point to the same memory location. |
- What are the main concepts of OOPs in Java?
The main OOPs concepts in Java are:
- Inheritance
- Polymorphism
- Abstraction
- Encapsulation
- What is a Class Variable?
Class variables also known as static variable are declared with the static keyword in a class, but outside a method, constructor or a block. There would only be one copy of each class variable per class, regardless of how many objects are created from it.
class Example {
public static int ctr = 0;
public Example() {
ctr++;
}
public static void main(String[] args) {
Example obj1 = new Example();
Example obj2 = new Example();
Example obj3 = new Example();
System.out.println("Number of objects created are " + Example.ctr);
}
}
Output
Number of objects created are 3
- How is an infinite loop declared in Java?
We will see how to make all three loops in Java an infinite one.
- for Loop
for (;;) { //body of the for loop... }
- while loop
while(true) { // body of the loop... }
- do...while loop
do { // body of the loop... }while(true);
- What if I write static public void instead of public static void?
The Java program will run successfully if you write a static public void main instead of the conventional public static void main. Java is flexible regarding the order of access modifiers, and both declarations are valid for the main method.
- What are the key differences between Java constructors and Java methods?
Java Constructor | Java Method |
A constructor is used to initialize the state of an object. | A method is used to expose the behavior of an object. |
A constructor must not have a return type. | A method must have a return type. |
The constructor is invoked implicitly. | The method is invoked explicitly. |
The Java compiler provides a default constructor if you don't have any constructor in a class. | The method is not provided by the compiler in any case. |
The constructor name must be the same as the class name. | The method name may or may not be the same as the class name. |
- When a byte datatype is used?
The byte data type can be useful for saving memory in large arrays, where memory savings matter. It can also be used in place of int, where its limits help to clarify your code.
- What is method overloading?
Method overloading in Java works for two or more methods or functions stored in the same class with the same name
- By Changing the number of arguments
- By Changing the data type of arguments
- What are the various access specifiers in Java?
There are four access specifiers/access modifiers in Java given below:
- Public: The classes, methods, or variables which are defined as public, can be accessed by any class or method.
- Protected: The protected methods and members can be accessed by the class of the same package, by the sub-class of this class, or within the same class.
- Default: these methods and classes are accessible within the package only. By default, all the classes, methods, and variables are of default scope.
- Private: The private class, methods, or variables can be accessed within the class only.
- What is a dot operator in Java?
Dot operator in Java is a syntactic element. It is used for three purposes:
- It separates a variable and method from a reference variable.
- It is also used to access classes and sub-packages from a package.
- It is also used to access the member of a package or a class.
Example
System.out.println("This is the main method");
import java.io.*;
Java Interview Questions and Answers for Intermediates
For intermediate-level Java developers, these interview questions will help you strengthen your understanding of key concepts such as input handling, exception management, OOP relationships, and object creation. They will also enhance your ability to write optimized and structured Java programs.
- In how many ways can you take input from the console?
We can do this in four ways in Java Online Compiler:
- Using Command line argument
class ScholarHat { public static void main(String[] args) { // Check if the length of the args array is greater than 0 if (args.length > 0) { System.out.println("The command line arguments are:"); // Iterating the args array and printing the command line arguments for (String value : args) System.out.println(value); } else System.out.println("No command line arguments found."); } }
- Compile the code in cmd using the below command
javac ScholarHat.java
- Run the program with command line arguments
java ScholarHat WelcometoScholarHat
Output
The command line arguments are: WelcometoScholarHat
- Compile the code in cmd using the below command
- Using Buffered Reader Class
import java.io.*; class ScholarHat { public static void main(String[] args) throws IOException { // Enter data using BufferedReader BufferedReader reader = new BufferedReader(new InputStreamReader(System.in)); System.out.println("Please enter some text:"); // Reading data using readLine String input = reader.readLine(); // Printing the read line System.out.println("You entered: " + input); } }
Output
Please enter some text: Hello, ScholarHat You entered: Hello, ScholarHat
- Using Console Class
public class ScholarHat { public static void main(String[] args) { // Using Console to input data from the user String x = System.console().readLine(); System.out.println("You entered string " + x); } }
Output
You entered string Hello, ScholarHat!
- Using Scanner Class
import java.util.Scanner; public class ScholarHat { public static void main(String[] args) { Scanner in = new Scanner(System.in); System.out.print("Enter a string: "); String str = in.nextLine(); System.out.println("You entered string " + str); } }
Output
Enter a string: Hello, ScholarHat You entered string Hello, ScholarHat
- What do we get in the JDK file?
JDK (Java Development Kit) is a package that contains various tools, Compilers, Java Runtime Environment, etc.
- JRE:The Java Runtime Environment contains a library of Java classes and a JVM. Java classes contain some predefined methods that help Java programs use features, build, and execute. For example, there is a system class in Java that contains the print-stream method, which allows us to print something on the console.
- JVM:The Java Virtual Machine is part of JRE that executes the Java program at the end. It is software that converts bytecode into machine-executable code to execute on hardware.
- What is the difference between the throw and throws keywords in Java?
- The throw keyword in Java is used to manually throw the exception to the calling method.
- The throws keyword in Java is used in the function definition to inform the calling method that this method throws the exception. So if you are calling, then you have to handle the exception.
class Main {
public static int divideByZero(int a, int b) throws ArithmeticException {
if (a == 0 || b == 0)
throw new ArithmeticException("Division by zero is not allowed");
return a / b;
}
public static void main(String[] args) {
try {
int result = divideByZero(10, 0);
System.out.println("Result: " + result);
} catch (ArithmeticException e) {
System.out.println("Exception caught: " + e.getMessage());
}
}
}
In the above Java code, the divideByZero()
method checks for division by zero and throws an ArithmeticException if either a or b is zero. In the main method, we catch the exception and print a message indicating that division by zero is not allowed.
Output
Enter a string:
Exception caught: Division by zero is not allowed
- What is the Is-A relationship in OOPs in Java?
In Java, inheritance is an is-a relationship. We can use inheritance only if there is an is-a relationship between two classes. For example,
In the above figure:
- A car is a Vehicle
- A bus is a Vehicle
- A truck is a Vehicle
- A bike is a Vehicle
Here, the Car class can inherit from the Vehicle class, the bus class can inherit from the Vehicle class, and so on.
- What do you understand by the term Vector in Java?
The Vector class is an implementation of the List interface with which you can create resizable arrays similar to the ArrayList class.
Syntax
Vector vector = new Vector<>();
- Vector can be imported using Java.util.Vector.
- Elements of the Vector can be accessed using index numbers.
- Vectors are synchronized in nature i.e. they only use a single thread ( only one process is performed at a particular time ).
- The vector contains many methods that are not part of the collections framework.
- What do you understand by constructor overloading in Java?
Constructor overloading in Java happens in Java when more than one constructor is declared inside a class but with different parameters. If an object in a class is created by using a new keyword, it generates a constructor in that class.
Example of Constructor Overloading in Java Playground
public class ScholarHat {
// function for adding two integers
void add(int a, int b) {
int sum = a + b;
System.out.println("Addition of two integers: " + sum);
}
// function for concatenating two strings
void add(String s1, String s2) {
String con_str = s1 + s2;
System.out.println("Concatenated strings: " + con_str);
}
public static void main(String args[]) {
ScholarHat obj = new ScholarHat();
// addition of two numbers
obj.add(10, 10);
// concatenation of two strings
obj.add("Operator ", "overloading ");
}
}
Output
Addition of two integer :20
Concatenated strings :Operator overloading
- Is it necessary that each try block must be followed by a catch block?
No, a catch block doesn't need to be present after a try block. A try block should be followed either by a catch block or by the finally block. If there are chances of more exceptions to occur, they should be declared using the throws clause of the method.
- What is the difference between a program and a process?
- A program can be defined as lines of code written to accomplish a particular task. Whereas the process can be defined as the programs which are under execution.
- A program doesn't execute directly by the CPU. First, the resources are allocated to the program, and when it is ready for execution, then it is a process.
- Differentiate between an object-oriented programming language and an object-based programming language.
Object-Oriented Programming Language | Object-Based Programming Language |
It covers concepts like inheritance, polymorphism, abstraction, etc. | The scope of object-based programming is limited to the usage of objects and encapsulation. |
It supports all the built-in objects | It doesn’t support all the built-in objects |
Examples: Java, C#, etc. | Examples: JavaScript, visual basics, etc. |
- Write a Java program to create and throw custom exceptions.
class ScholarHat {
public static void main(String[] args) throws CustomException {
// Throwing the custom exception by passing the message
throw new CustomException("This is an example of custom exception");
}
}
// Creating Custom Exception Class
class CustomException extends Exception {
// Defining Constructor to throw exception message
public CustomException(String message) {
super(message);
}
}
- What happens if there are multiple main methods inside one class in Java?
The program will not compile. The Java Compiler will give a warning that the method has been already defined inside the class.
- How is the creation of a string using new() different from that of a literal?
- When a String is created using the new() keyword, a new String object is created in memory every time, even though the content of the String is the same as an existing String. It means that two String objects with the same content will be stored in different memory locations, and comparing them using the == operator will return false, even if their contents are the same.
Example
public class StringComparison { public static void main(String[] args) { String str1 = new String("Hello ScholarHat"); String str2 = new String("Hello ScholarHat"); // Comparing references of str1 and str2 System.out.println(str1 == str2); } }
Output
false
- When a String is created using a literal, the JVM checks if a String with the same content already exists in the String pool. If a String with the same content already exists in the String pool, a reference to that String object is returned instead of creating a new String object. This means that two String literals with the same content will reference the same String object in the memory, and comparing them using the == operator will return true.
Example
public class StringComparison { public static void main(String[] args) { String str1 = new String("Hello ScholarHat"); String str2 = new String("Hello ScholarHat"); // Comparing references of str1 and str2 System.out.println(str1 == str2); } }
Output
true
For more information, click on Strings in Java
- Is it possible to call a constructor of a class inside another constructor?
Yes, this is possible and this can be termed as constructor chaining and can be achieved using this().
- Are int array[] and int[] array different or the same?
In Java, both int array[] and int[] array are valid syntaxes for declaring an array of integers.
int arr[] //C-Style syntax to declare an array.
int[] arr //Java-Style syntax to declare an array.
- What are the different ways to create objects in Java?
- Using
new
keyword - Using
new
instance - Using
clone()
method - Using
deserialization
- Using the
newInstance()
method of the constructor class
To get detailed insights on all these methods,refer to Classes and Objects in Java OOPs
Java interview questions for 3 years of experience
For professionals with 3 years of experience, these Java interview questions will help you solidify your understanding of advanced concepts like the final and super keywords, exception handling, collections, and design patterns. They will also improve your ability to explain core Java principles with clarity.
36. What is the purpose of the final
keyword in Java?
final
the keyword is used to declare constants and prevent method overriding and inheritance.- Final Variable: Its value cannot be changed.
- Final Method: Cannot be overridden.
- Final Class: Cannot be subclassed.
37. Explain the difference between ArrayList
and LinkedList
.
- ArrayList: Better for random access as it uses a dynamic array.
- LinkedList: Better for frequent insertions and deletions as it uses a doubly linked list.
38. What is the purpose of the super
keyword?
super
keyword refers to the immediate parent class object and is used to:- Call the parent class constructor.
- Access parent class methods and variables.
39. What is a marker interface?
Serializable
, Cloneable
.40. What is a Singleton Class?
41. What is the difference between checked
and unchecked
exceptions?
- Checked: Must be handled during compile time (e.g.,
IOException
). - Unchecked: Occurs at runtime (e.g.,
NullPointerException
).
42. What is the difference between ==
and .equals()
in Java?
==
compares references..equals()
compares values (overridden for content comparison in classes likeString
).
43. What is a Java Package, and why is it used?
44. How does try-catch-finally
work in Java?
try:
Code to monitor for exceptions.catch:
Handles exceptions.finally:
Always executes (used for cleanup).
45. What is the this
keyword in Java?
- Differentiates between local and instance variables.
- Calls another constructor in the same class.
Java interview questions for 5 years of experience
For professionals with 5 years of experience, these Java interview questions will help you deepen your expertise in advanced topics like Java Reflection API, Garbage Collection, Functional Interfaces, and the Fork/Join framework. They will also enhance your ability to write efficient and optimized Java applications.
46. Explain the concept of Java Reflection API.
47. What are annotations in Java?
@Override
, @Deprecated
.48. How does Garbage Collection work in Java?
49. What is a Soft Reference in Java?
50. What is a deadlock, and how can it be avoided?
- Acquiring locks in the same order.
- Using timeouts when acquiring locks.
51. What are Functional Interfaces in Java?
Runnable
, Callable
.52. What is Java Stream API?
53. What is the difference between Comparable and Comparator?
Comparable:
Defines natural ordering (compareTo()
).Comparator:
Defines custom ordering (compare()
).
54. What is method reference in Java?
ClassName::methodName
.55. How does the Fork/Join framework work?
Java interview questions for 10 years of experience
For professionals with 10 years of experience, these Java interview questions will help you master advanced topics like concurrency, design patterns, Java NIO, microservices, and reactive programming. They will also enhance your ability to architect scalable and high-performance Java applications.
56. What is the significance of the volatile
keyword?
volatile
keyword ensures that a variable's value is always read from the main memory and not from the thread's local cache.57. What are the design patterns you have used in Java?
58. How does the Java Memory Model handle concurrency?
59. What is the difference between ThreadLocal and synchronized?
ThreadLocal:
Provides thread-local variables.synchronized:
Ensures thread-safe access to shared resources.
60. Explain the working of Java NIO.
61. How do you implement microservices using Java?
62. What is the difference between fail-fast and fail-safe iterators?
- Fail-fast: Throws
ConcurrentModificationException
if the collection is modified during iteration. - Fail-safe: Allows modification as they work on a copy of the collection.
63. What is Reactive Programming in Java?
64. What is the difference between REST and SOAP?
- REST: Lightweight, uses JSON, and works over HTTP.
- SOAP: Protocol-based, uses XML, and supports advanced security features.
65. What are Java Modules, and why are they used?
Advanced Java Interview Questions and Answers
For those preparing for advanced Java interviews, these questions will help you strengthen your understanding of key concepts such as polymorphism, thread lifecycle, abstract classes vs. interfaces, and memory management. They will also enhance your ability to write efficient and optimized Java applications.
66. What are the differences between abstract class and interface?
Abstract Class | Interface |
Both abstract and non-abstract methods may be found in an abstract class. | The interface contains only abstract methods. |
The abstract class supports final methods. | An interface does not support final methods. |
The abstract class does not support multiple inheritance. | The interface supports multiple inheritance. |
Abstract keyword is used to declare an abstract class. | Interface keyword is used to declare an interface. |
Extend keyword is used to extend an abstract Class. | Interface keyword is used to implement an interface. |
Abstract class has members such as protected, private, etc. | All class members are public by default. |
67. Explain System.out.println().
System.out.println() prints the message on the console.
System
- It is a class present injava.lang package
.out
is the static variable of typePrintStream
class present in theSystem class
.println()
is the method present in the PrintStream class.
68. Can this keyword be used to refer to static members?
Yes, it is possible to use this keyword to refer to static members because this is just a reference variable that refers to the current class object.
Example in Java Online Editor
public class ScholarHat {
static int i = 50;
public ScholarHat() {
System.out.println(this.i);
}
public static void main(String[] args) {
ScholarHat t = new ScholarHat();
}
}
Output
50
69. Explain super in Java.
Example
class ScholarHat {
ScholarHat() {
System.out.println("ScholarHat class is created");
}
}
class Student extends ScholarHat {
Student() {
System.out.println("Student class is created");
}
}
public class Test {
public static void main(String[] args) {
Student s = new Student();
}
}
Output
ScholarHat class is created
Student class is created
70. What is runtime polymorphism or dynamic method dispatch?
Runtime polymorphism, also known as dynamic method dispatch, is a mechanism that resolves method overriding at runtime. Polymorphism allows a subclass to provide a specific implementation of a method that already exists in its superclass, maintaining the same method name, parameters, and return type.
When a method is called using a superclass reference, Java determines which version (superclass or subclass) should execute based on the actual object being referenced at runtime. Since the method execution is decided dynamically, this behavior is called dynamic method dispatch, demonstrating polymorphism in action.
71. How is the new operator different from the newInstance() operator in Java?
Both ‘new’ and ‘newInstance()’ operators are used to create objects.
The difference is, that when we already know the class name for which we have to create the object we use a new operator. If we don’t know the class name for which we need to create the object, we get the class name from the command line argument, the database, or the file. In all such cases, we use the newInstance() operator.
72. Explain the Java thread lifecycle.
- New—When the thread instance is created and the start() method has not been invoked, the thread is considered to be alive and hence in the NEW state.
- Runnable—Once the start() method is invoked and before the run JVM calls () method, the thread is said to be in a RUNNABLE (ready to run) state. This state can also be entered from the thread's Waiting or Sleeping state.
- Run – When the run() method has been invoked and the thread starts its execution, the thread is said to be RUNNING.
- Blocked/Waiting – When the thread is not able to run despite being alive, the thread is said to be in a NON-RUNNABLE state. Ideally, after some time, the thread should go to a runnable state.
- A thread is said to be in a blocked state if it wants to enter synchronized code but it is unable to as another thread is operating in that synchronized block on the same object. The first thread has to wait until the other thread exits the synchronized block.
- A thread is said to be waiting if it is waiting for the signal to execute from another thread, i.e. it waits for work until the signal is received.
- Terminated – Once run() method execution is completed, the thread is said to enter the TERMINATED stage and is considered dead.
73. State the differences between method overloading and overriding.
Method Overloading | Method Overriding |
Method overloading increases the readability of the program. | Method overriding provides the specific implementation of the method that is already provided by its superclass. |
Method overloading occurs within the class. | Method overriding occurs in two classes that have an Is-A relationship between them. |
The parameters must be different here. | The parameters must be the same. |
74. When can an object reference be cast to an interface reference?
An object reference can be cast to an interface reference in Java when the object's class implements that interface.
Example
interface Animal {
void sound();
}
class Dog implements Animal {
public void sound() {
System.out.println("Woof!");
}
}
public class Main {
public static void main(String[] args) {
// Creating a Dog object
Dog dog = new Dog();
// Casting Dog object to Animal interface reference
Animal animal = (Animal) dog;
// Calling the sound() method through the Animal interface reference
animal.sound();
}
}
Output
Woof
In the above code written in Java Online Editor, the Dog class implements the Animal interface, so it's safe to cast a Dog object to an Animal interface reference. This allows us to call the sound() method defined in the Animal interface through the Animal interface reference.
75. What do you understand by the jagged array?
A jagged array is an array of arrays such that member arrays can be of different sizes, i.e., we can create a 2-D array but with a variable number of columns in each row.
Example
int[][] arr = new int[][] {
{1, 2, 3},
{4, 5},
{6, 7, 8, 9}
};
76. Will the finally block be executed if the code System.exit(0) is written at the end of the try block?
No, if System.exit(0) is called at the end of the try block, the finally block will not be executed. The System.exit(0) method abruptly terminates the JVM (Java Virtual Machine) with a status code of 0.
77. What if we import the same package/class twice? Will the JVM load the package twice at runtime?
In Java, we can import the same package/class twice. However, regardless of the number of times you import, the JVM loads the class only once.
Example
import java.util.ArrayList;
import java.util.List;
public class Main {
public static void main(String[] args) {
// Create a list
List list = new ArrayList<>();
// Add elements to the list
list.add("Apple");
list.add("Banana");
list.add("Orange");
// Print the list
System.out.println("List: " + list);
}
}
In this example, the ArrayList class is imported twice, once explicitly (import java.util.ArrayList;) and once indirectly through importing the java.util.List interface (import java.util.List;).
Output
List: [Apple, Banana, Orange]
78. What are the differences between StringBuffer and StringBuilder?
StringBuffer | StringBuilder |
StringBuffer is synchronized, i.e., thread-safe. It means two threads can't call the methods of StringBuffer simultaneously. | StringBuilder is non-synchronized,i.e., not thread-safe. This means two threads can call its methods simultaneously. |
The String class overrides the equals() method of the Object class. So you can compare the contents of two strings by the equals() method. | StringBuilder is more efficient than StringBuffer. |
79. In what ways is inheritance in C++ different from Java?
Inheritance in Java | |
There is multiple inheritance possible in C++ | Java doesn’t support multiple inheritances. |
When a class is created in C++, it doesn’t inherit from the object class, instead exists on its own. | Java is always said to have a single inheritance as all the classes inherit in one or the other way from the object class. |
80. Are virtual functions available in Java?
No, like virtual functions in C++, virtual functions aren't a feature of Java. The functionality is achieved through method overriding and dynamic method dispatch.
Read More:
- Top 50 Core Java Interview Questions
- Top 50 Java MCQ Questions
- Java Multithreading Interview Questions and Answers
- Top 50+ Java 8 Interview Questions & Answers
- Top 50+ Java 8 Interview Questions & Answers
Summary
The Java Interview Questions for 10 Years Experience article covers 80 advanced questions to help you master core Java, multithreading, design patterns, JVM internals, performance tuning, and best practices. It provides in-depth explanations and real-world scenarios to boost your confidence in senior-level interviews. Whether you're preparing for an architect or lead developer role, these questions will enhance your expertise. Want to excel in software architecture? Explore the Software Architecture Design Training to build scalable and efficient systems.
Need to refresh your Java fundamentals? Enroll in this Free Java Course and strengthen your core concepts!
Download this PDF Now - JAVA Interview Questions and Answers PDF By ScholarHat |
Java interview questions for 10 years experience - Test Your Knowledge!
Q 1: What are the key differences between HashMap, LinkedHashMap, and TreeMap?
- (a) HashMap maintains insertion order
- (b) LinkedHashMap does not maintain order
- (c) TreeMap is sorted based on natural order or comparator
- (d) HashMap allows duplicate keys
Q 2: How does the Java Garbage Collector work?
- (a) It manually deletes objects
- (b) It removes objects that have no active references
- (c) It runs only when memory is full
- (d) It requires explicit invocation
Q 3: What are the key differences between Checked and Unchecked Exceptions?
- (a) Checked exceptions must be handled at compile-time
- (b) Unchecked exceptions require explicit handling
- (c) Checked exceptions are part of java.lang package
- (d) Unchecked exceptions extend the Throwable class directly
Q 4: What is the difference between ExecutorService and ForkJoinPool?
- (a) ExecutorService is for simple multi-threading
- (b) ForkJoinPool is optimized for recursive task execution
- (c) ExecutorService does not support work stealing
- (d) ForkJoinPool does not support parallel execution
Q 5: What is the significance of Java Streams API?
- (a) Streams process data in parallel
- (b) Streams mutate collections
- (c) Streams operate only on arrays
- (d) Streams do not support lazy evaluation
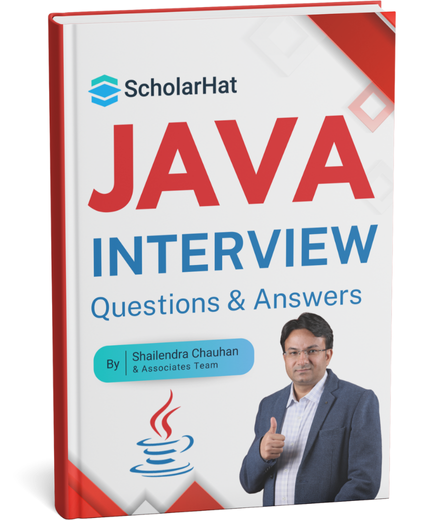
Crack Your Next Java Interview – Grab the Free Expert eBook!
Java Interview Questions and Answers Book Unlock expert-level Java interview preparation with our exclusive eBook! Get instant access to a curated collection of real-world interview questions, detailed answers, and professional insights — all designed to help you succeed.
No downloads needed — just quick, free access to the ultimate guide for Java interviews. Start your preparation today and move one step closer to your dream job!.